Free PLC course outline
- Lesson #1: What Is It and Why Should Every PLC Programmer Know It? Part #1
- Lesson #2: How to install Codesys? Part #2
- Lesson #3: Write your first program in Codesys: Structured Text – Part #3
- Lesson #4: How to Create a Codesys Visualization in an Application? – Part #4
- Lesson #5: Introduction to Variables in CODESYS – Part #5
- Lesson #6: Data Structures in CODESYS: Practical Use of Arrays and Structures – Part #6
- Lesson #7: Advanced Data Types. Enumerations and Local vs. Global Variables – Part #7
- Lesson #8: Operators – Introduction and Practical Applications – Part #8
- Lesson #9: Program Flow Control: IF, CASE, and Loops – Part #9
In PLC programming, operators play a crucial role in executing calculations, logical conditions, and data manipulations. CODESYS provides a variety of operators that allow developers to efficiently control automation processes. This section explores different types of operators, their syntax, and real-world applications.
Introduction to Operators
Operators in CODESYS are used to perform arithmetic operations, logical evaluations, and value comparisons. These operators can be utilized in both graphical languages (Ladder Logic – LAD) and text-based languages (Structured Text – ST).
One of the fundamental differences between Ladder Logic (LAD) and Structured Text (ST) is the direction of execution. In LAD, execution occurs from left to right, resembling an electrical circuit, whereas in ST, operations are written in a sequential manner, following standard programming logic.
Assignment Operator (:=)
Understanding the Assignment Operator in ST
The assignment operator (:=) is used to assign a value or result of an operation to a variable.
Example 1: Assigning a Value
xLampStart := xButton;
In this case, the value of xButton is assigned to xLampStart. When xButton is TRUE, xLampStart will also be TRUE.
Comparison: LAD vs. ST
In LAD, this same logic is implemented using a normally open (xButton) contact connected to a coil (xLampStart).
- Open LADDER_PRG and add a new network.
- Insert a Normally Open Contact (xButton).
- Add a Coil (xLampStart).
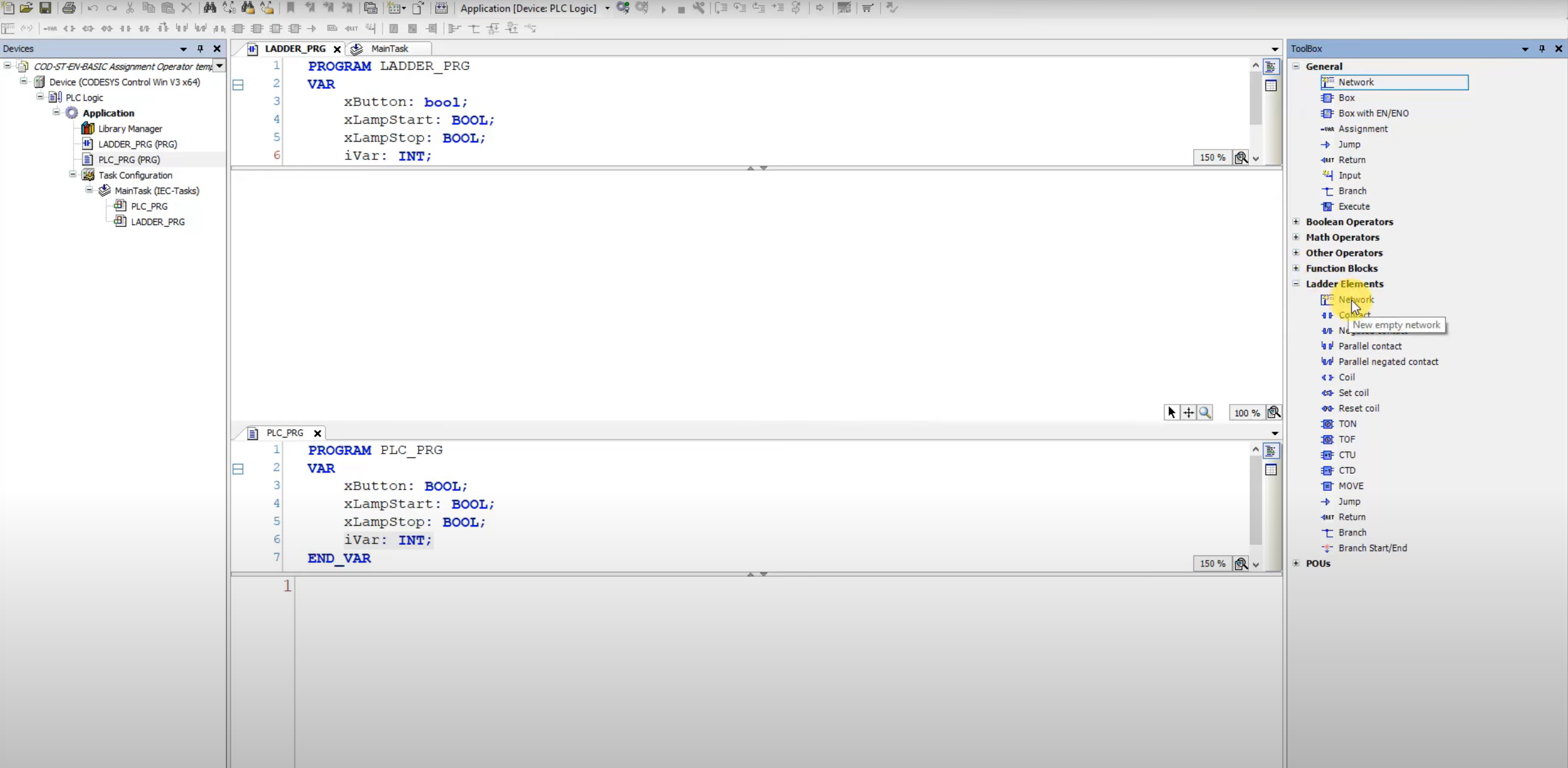
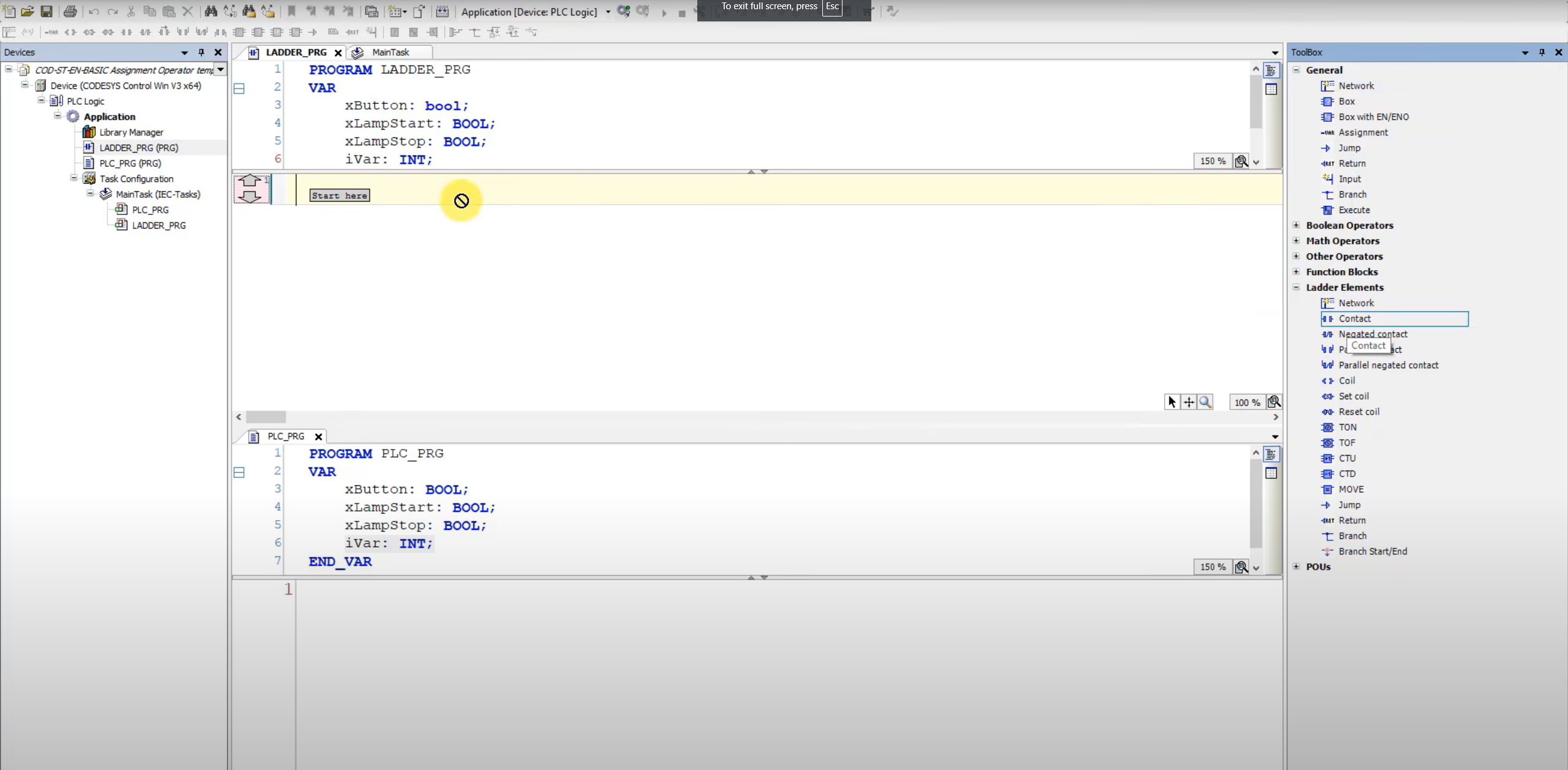
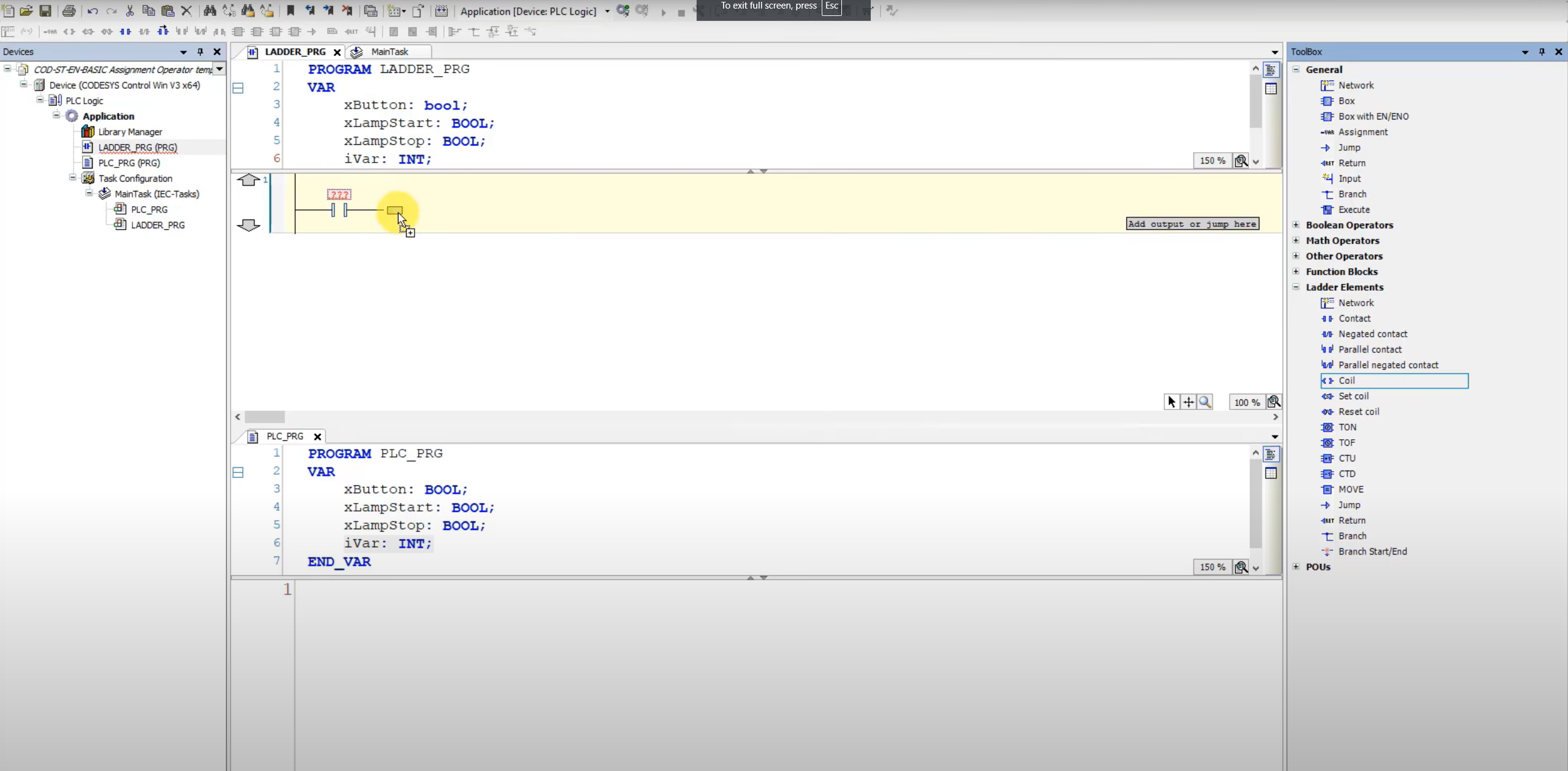

In ST, this logic is expressed concisely in a single line:
xLampStart := xButton;
Using the Assignment Operator with Logical Operations
The assignment operator can also work with logical expressions, such as negation (NOT).
Example 2: Using NOT Operator
xLampStop := NOT xLampStart;
Here, xLampStop will be TRUE when xLampStart is FALSE, ensuring proper control over the lamp state.
In LAD, the same logic would require inserting a Normally Closed Contact (xLampStart) followed by a Coil (xLampStop).
Move Operations and Assignments
In LAD, arithmetic operations require a MOVE instruction to transfer calculated values.
Example 3: Move Operation in LAD
- Insert a MOVE function.
- Input 10 + 20 in the source field.
- Assign the result to iVar.
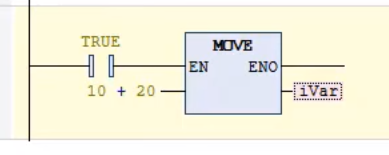
In ST, the same operation is expressed in a single line:
iVar := 10 + 20;
This approach makes ST more readable and efficient for handling complex computations.
Next Steps
This section covered the assignment operator and its comparison between LAD and ST. In the next section, we will explore arithmetic operators, their functions, and practical use cases in automation programming.
Arithmetic Operators in CODESYS: Usage and Best Practices
Arithmetic operators play a fundamental role in PLC programming, allowing developers to perform mathematical calculations in automation processes. Understanding how to use these operators correctly ensures accurate computations and efficient program execution.
Basic Arithmetic Operators in CODESYS
The basic arithmetic operators in CODESYS include:
- Addition (+) – Adds two numbers.
- Subtraction (-) – Subtracts one number from another.
- Multiplication (*) – Multiplies two numbers.
- Division (/) – Divides one number by another.
- Exponentiation (XPT) – Raises a number to a power.
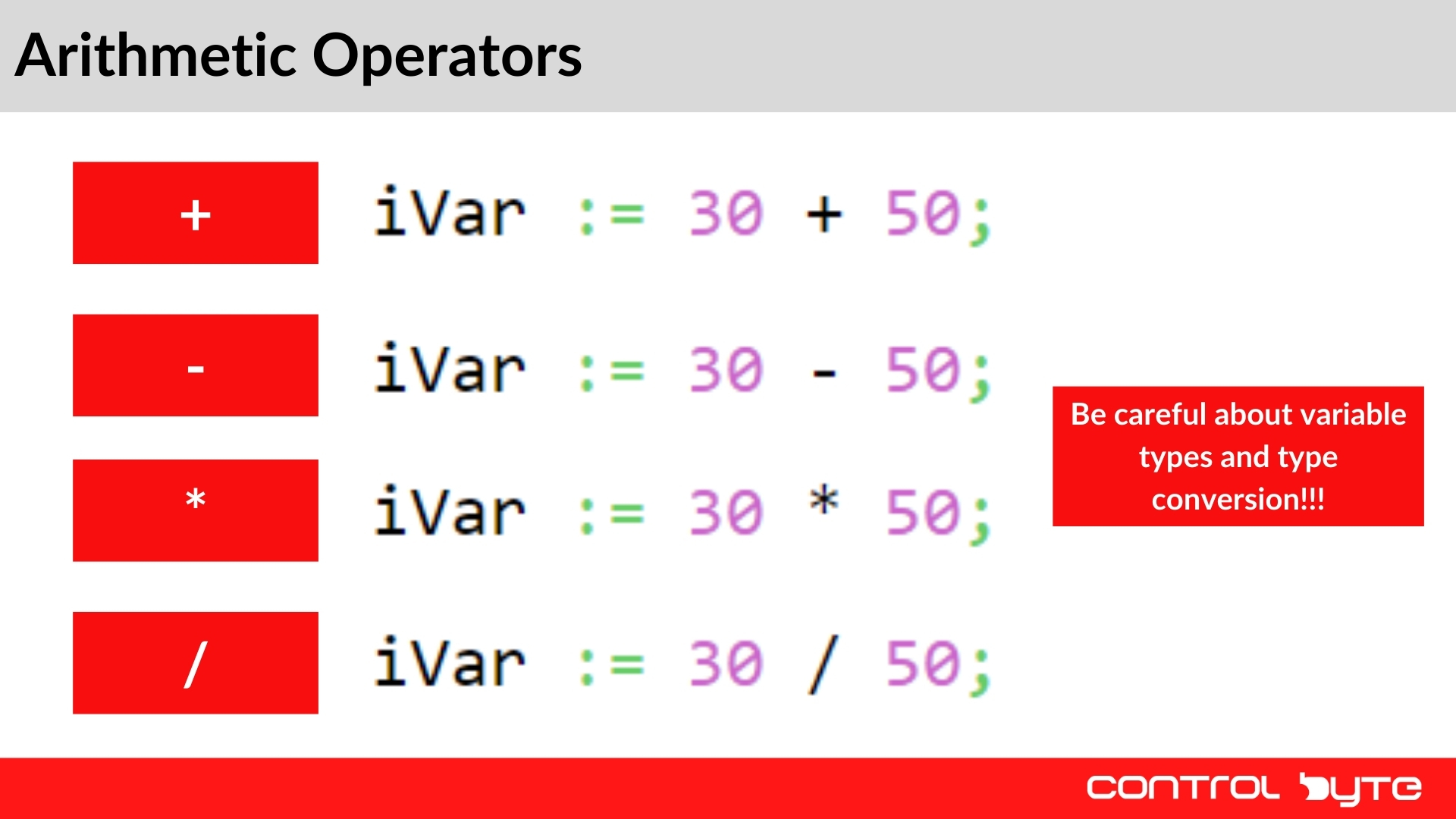
Performing Arithmetic Calculations
Let’s start with a simple addition operation.
VAR
iVar : INT;
END_VAR
iVar := 10 + 25;
In this example, iVar stores the result of 10 + 25, which is 35.
Executing the Program
To test the program in the PLC simulator:
- Log into the simulator (ALT + F8).
- Start the PLC execution (F5).
- Observe the output result.

Using Subtraction (–) Operator
iVar := 25 - 8;
Here, iVar stores 17 as the result of subtraction.
Important Note: If the same variable is used for multiple operations, the last operation executed determines the final value of the variable.
Handling Division (/) and Type Conversion
One of the common mistakes when using division (/) is not considering variable types.
Example 1: Integer Division
VAR
iResult : INT;
END_VAR
iResult := 5 / 3;
Since iResult is an integer, the fractional part is lost, and the stored result will be 1 instead of 1.666.
Example 2: Floating-Point Division
To obtain a precise result, a floating-point variable (REAL) should be used:
VAR
rResult : REAL;
END_VAR
rResult := 5.0 / 3.0;

Now, rResult stores 1.666, preserving decimal precision.
Using Multiplication (*)
iResult := 2 * 10;
This operation multiplies the value of iResult by 10.

Using Exponentiation (XPT)
The EXPT operator allows raising numbers to a power.
VAR
lrResult : LREAL;
Base : REAL := 2;
Exponent : REAL := 3;
END_VAR
lrResult := XPT(Base, Exponent);
In this case, lrResult stores 8, since 2 raised to the power of 3 equals 8.

Finding Help in CODESYS
To explore more details about operators, developers can:
Access CODESYS Online Help to find examples and explanations.
Hover over the operator and press F1.
Logical Operators in CODESYS: Implementation and Applications
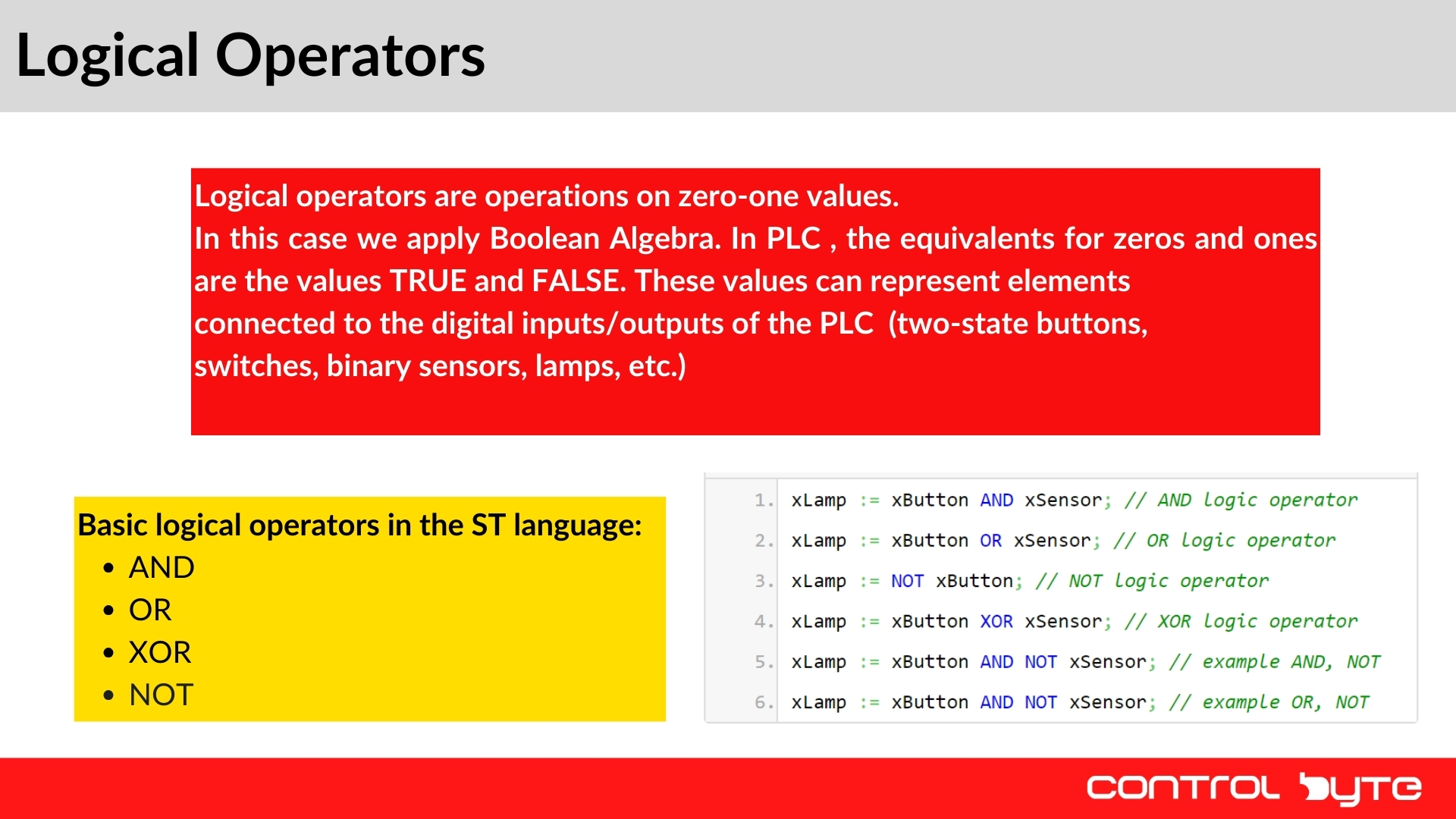
Logical operators in CODESYS play a crucial role in Boolean algebra, allowing developers to create conditional logic for controlling automation processes. These operators evaluate conditions and return either TRUE (1) or FALSE (0), which are fundamental in decision-making processes in PLC programming.
Basic Logical Operators in CODESYS
The fundamental logical operators include:
- AND (AND) – Returns TRUE if both conditions are TRUE.
- OR (OR) – Returns TRUE if at least one condition is TRUE.
- NOT (NOT) – Inverts a Boolean value.
- Exclusive OR (XOR) – Returns TRUE if exactly one of the conditions is TRUE.
Logical operators can be applied to Boolean variables, bitwise operations, and logical conditions.
Using the AND Operator
The AND operator requires both input conditions to be TRUE for the output to be TRUE.
Example: AND Operator in CODESYS
VAR
xButton1 : BOOL;
xButton2 : BOOL;
xLamp1 : BOOL;
END_VAR
xLamp1 := xButton1 AND xButton2;
Here, xLamp1 turns ON only when both xButton1 and xButton2 are pressed.
Testing in CODESYS Simulator
- Log in to the PLC simulator.
- Set xButton1 to TRUE → The lamp remains OFF.
- Set xButton2 to TRUE → The lamp now turns ON.
The truth table for the AND operation:
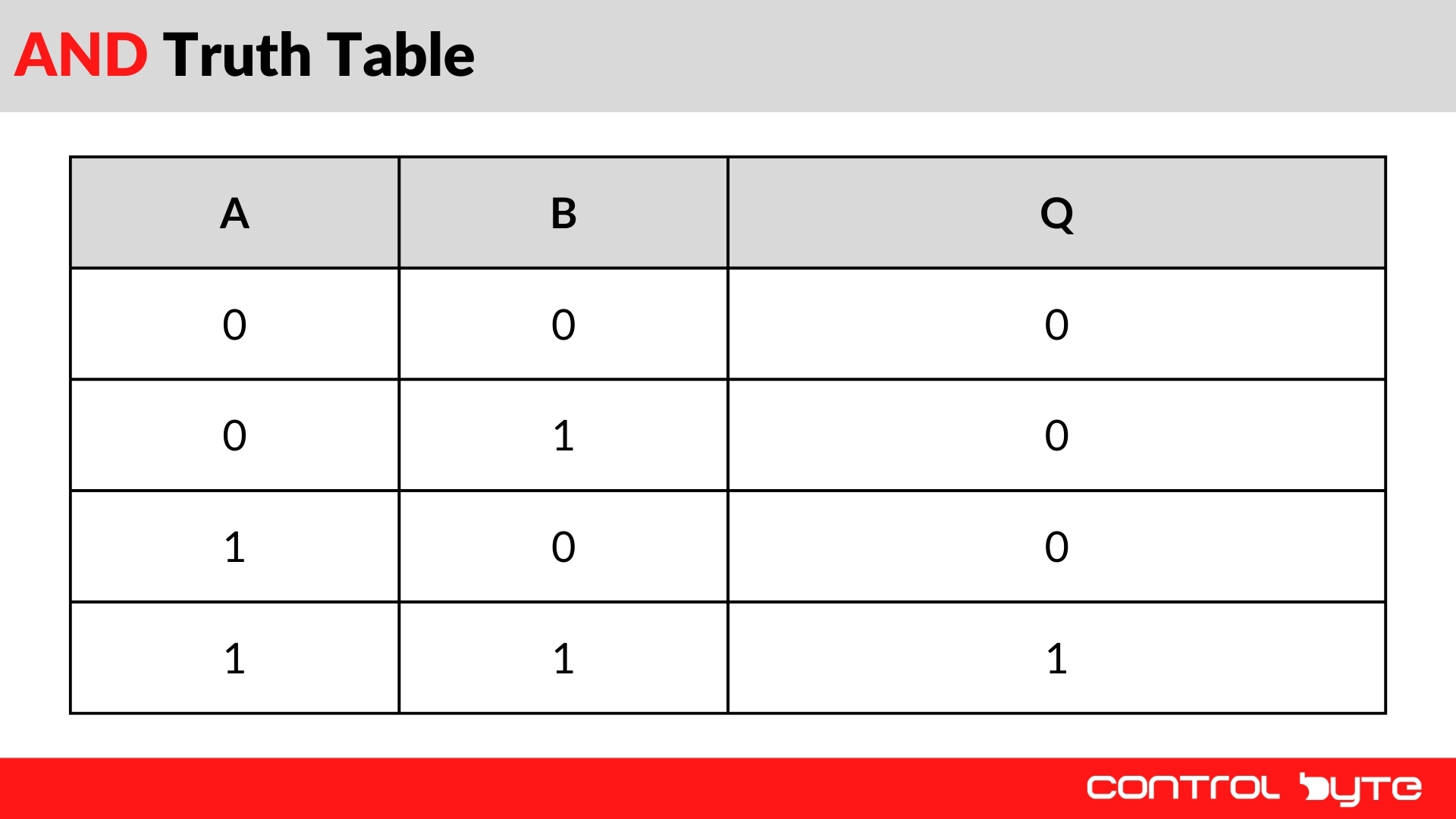
Using the OR Operator
The OR operator requires at least one condition to be TRUE for the output to be TRUE.
Example: OR Operator in CODESYS
xLamp2 := xButton1 OR xButton2;
Now, xLamp2 turns ON if either xButton1 or xButton2 is pressed.
Truth table for OR operation:
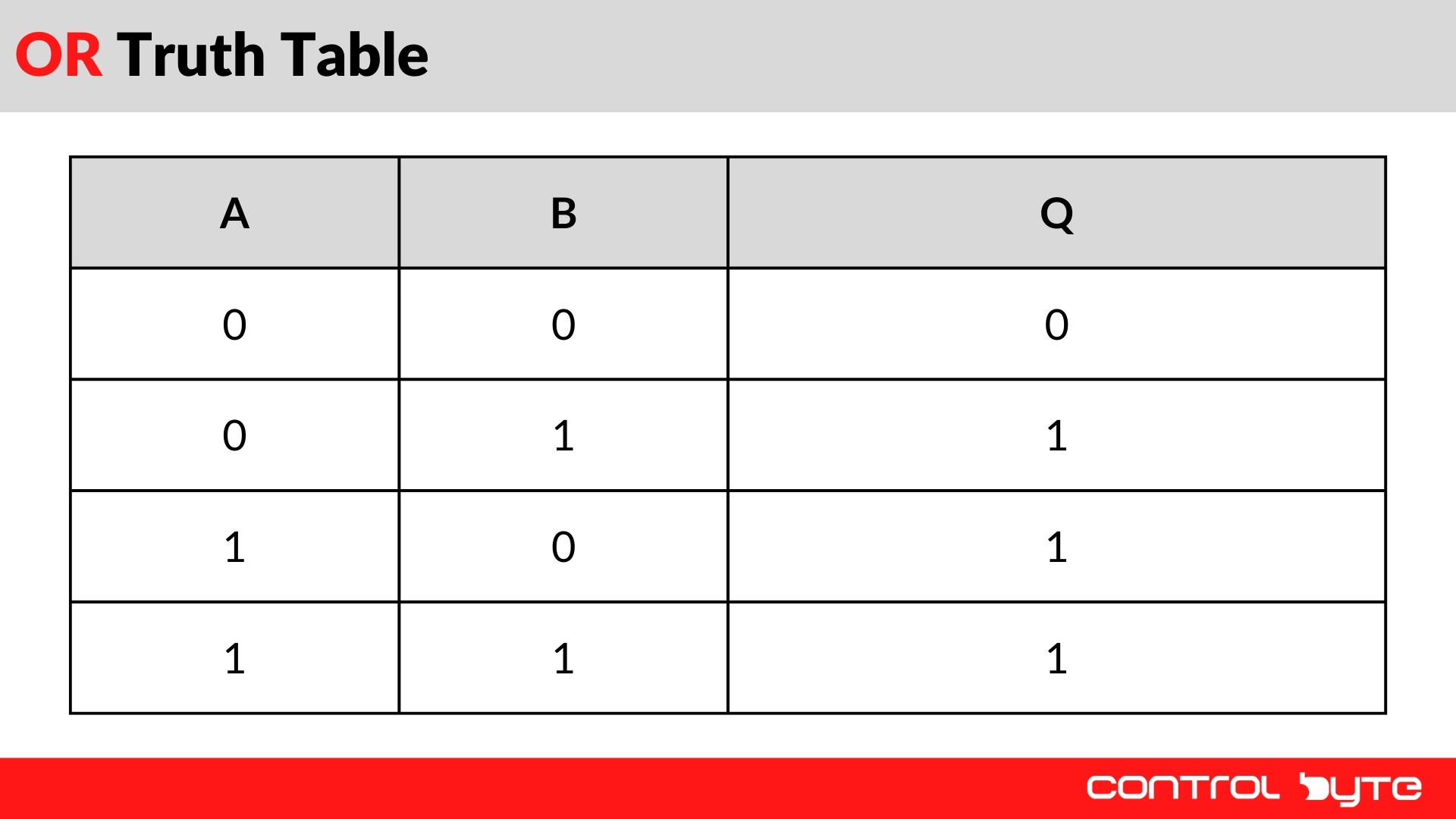
Using the NOT Operator
The NOT operator inverts a Boolean value, turning TRUE into FALSE and vice versa.
Example: NOT Operator in CODESYS
xLamp3 := NOT xButton1;
If xButton1 is FALSE, xLamp3 will be TRUE.
Truth table for NOT operation:
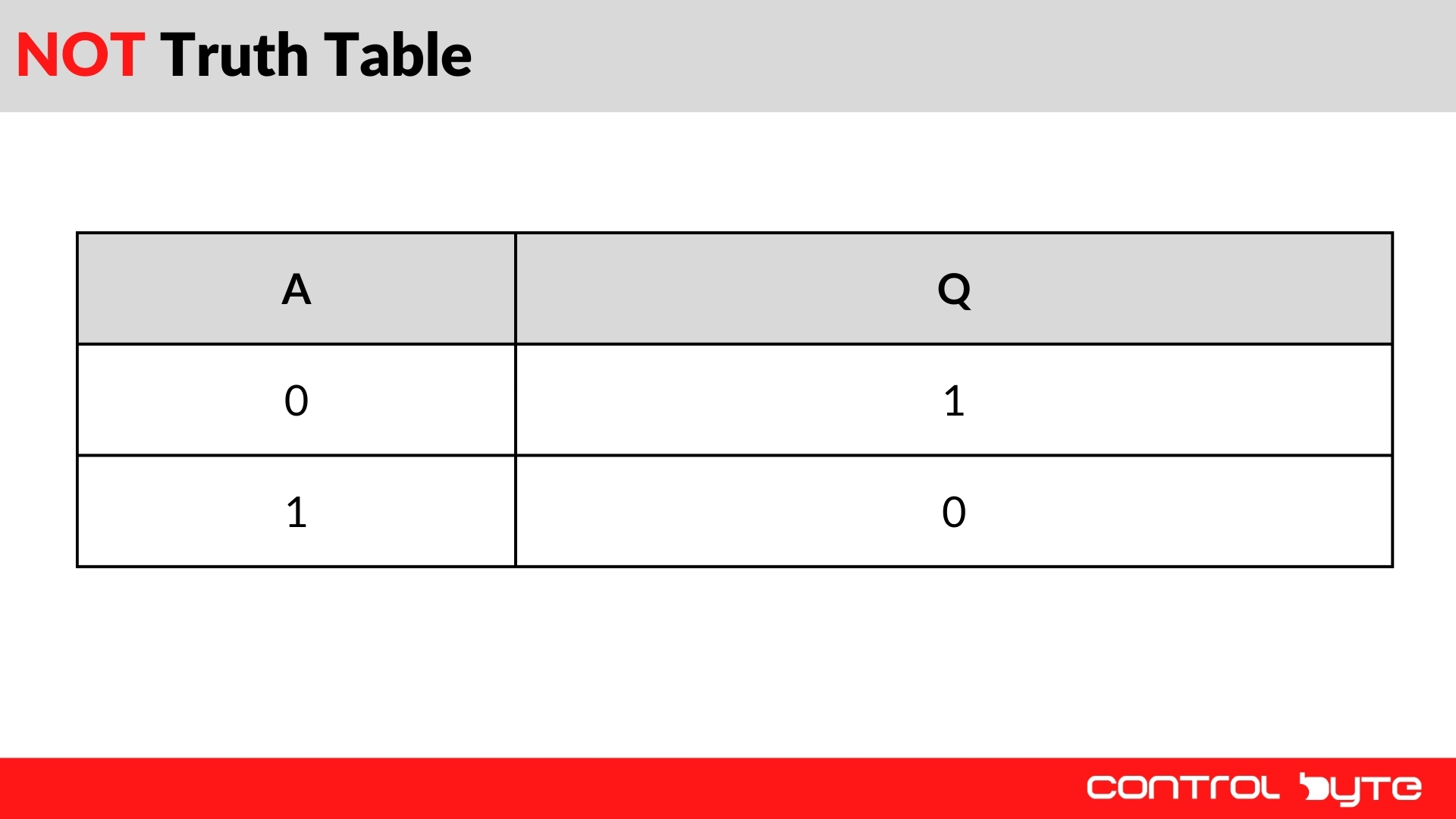
Using the XOR (Exclusive OR) Operator
The XOR operator returns TRUE only when one condition is TRUE and the other is FALSE.
Example: XOR Operator in CODESYS
xLamp4 := xButton1 XOR xButton2;
If only one of the buttons is pressed, the lamp turns ON.
Truth table for XOR operation:
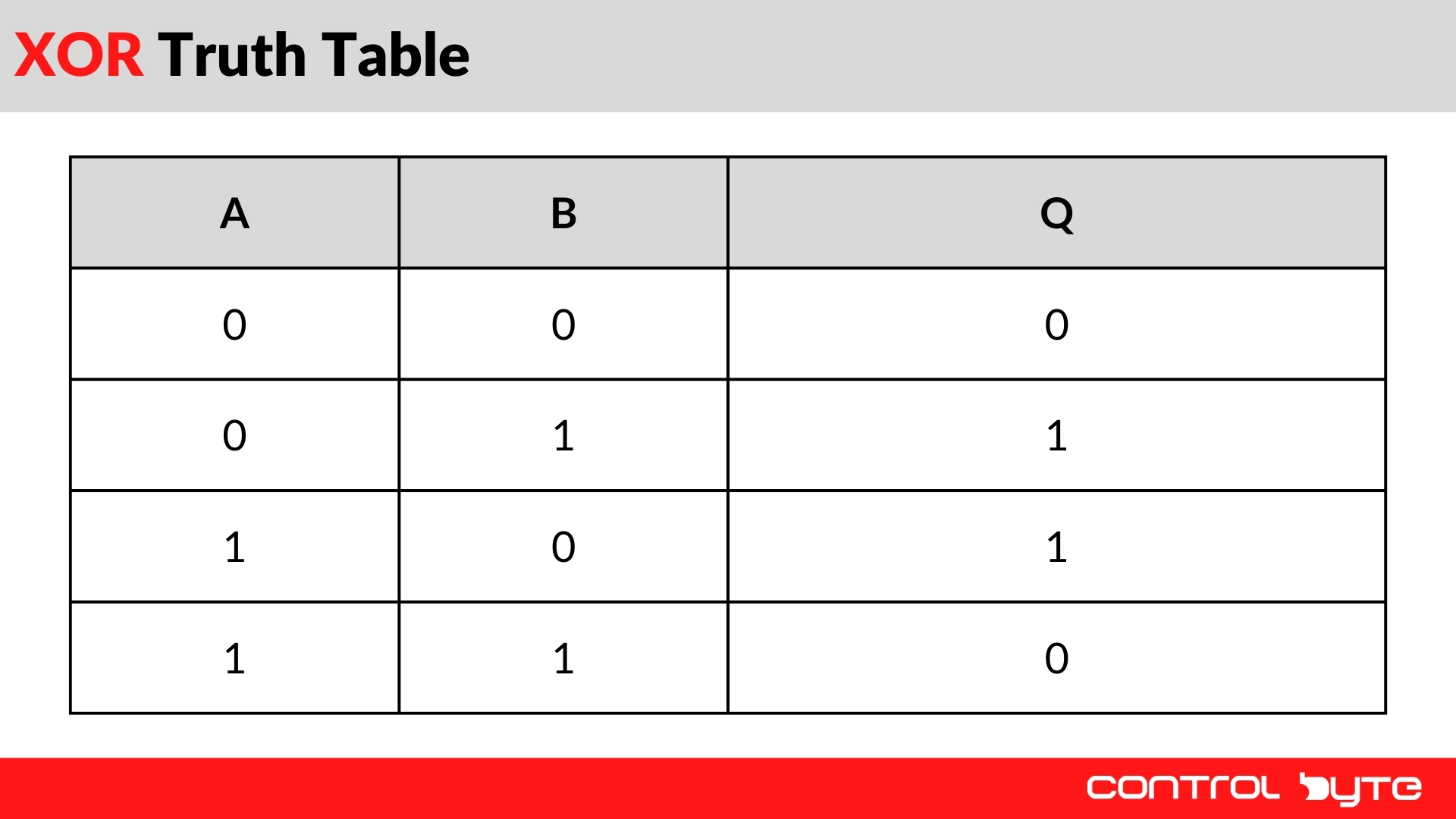
Combining Logical Operators
Logical operators can be combined to create more sophisticated conditions. For example:
xLamp5 := (xButton1 AND xButton2) OR (NOT xButton3);
This means xLamp5 will turn ON if:
- Both xButton1 and xButton2 are TRUE, OR
- xButton3 is FALSE
Operators in CODESYS: Logical and Comparison Operators
Logical and comparison operators in CODESYS are essential for decision-making processes in PLC programming. They allow developers to evaluate conditions, control program flow, and compare values, making automation systems more efficient and flexible.
Logical Operators in CODESYS
Logical operators evaluate Boolean expressions and return either TRUE or FALSE. The fundamental logical operators include:
- AND (AND) – Returns TRUE if both conditions are TRUE.
- OR (OR) – Returns TRUE if at least one condition is TRUE.
- NOT (NOT) – Inverts a Boolean value.
- Exclusive OR (XOR) – Returns TRUE if exactly one of the conditions is TRUE.
Example: Combining Logical Operators
xLamp5 := (xButton1 AND xButton2) OR (NOT xButton3);
This means xLamp5 will turn ON if:
- Both xButton1 and xButton2 are TRUE, OR
- xButton3 is FALSE
Comparison Operators in CODESYS
Comparison operators allow evaluating numerical conditions and are commonly used for control logic.
Basic Comparison Operators
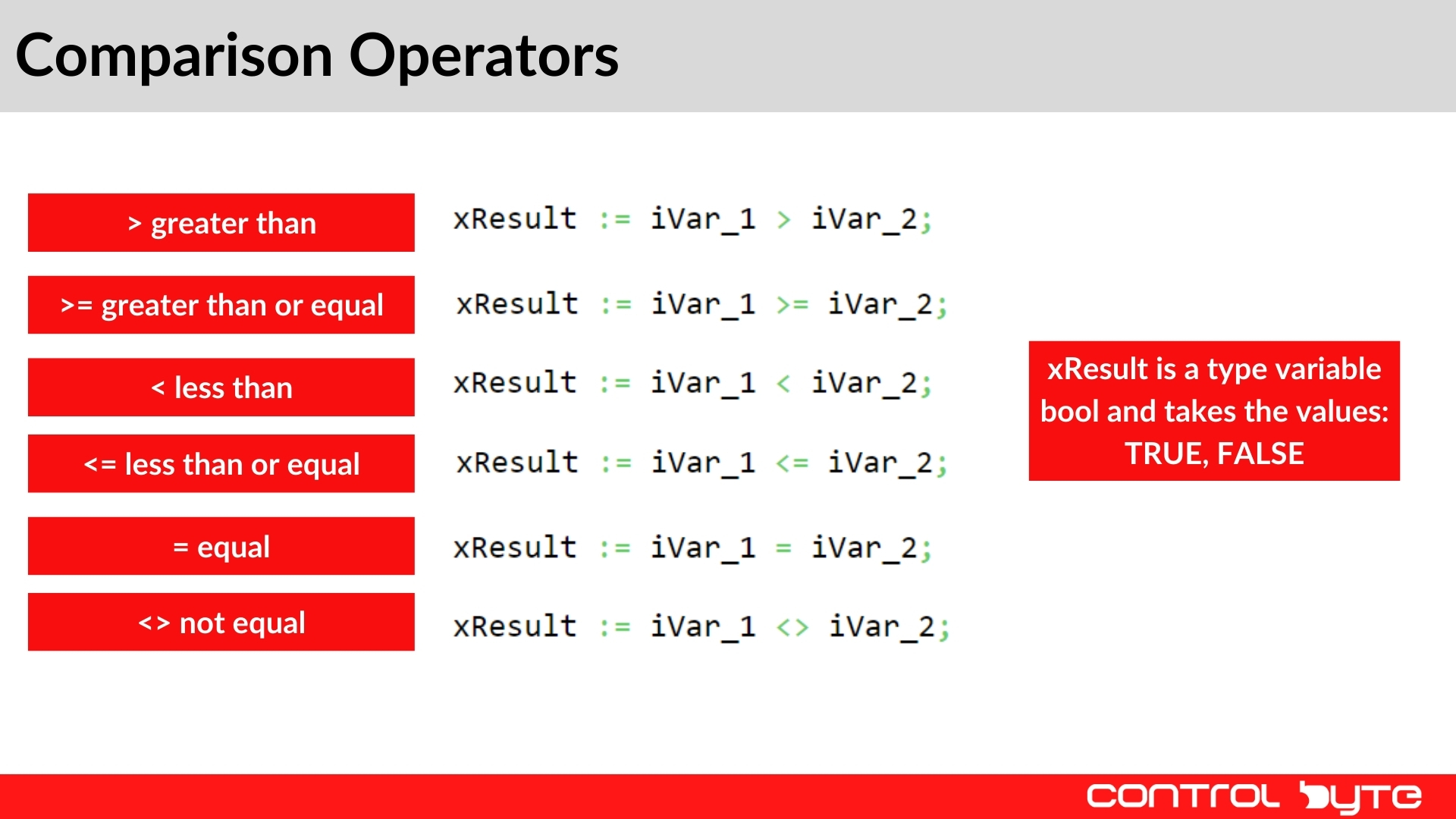
Example: Using Comparison Operators in CODESYS
VAR
iA : INT := 10;
iB : INT := 20;
xResult : BOOL;
END_VAR
xResult := iA < iB; // Returns TRUE
Testing Comparison Operators in PLC Simulator
- Log into the PLC simulator.
- Change the values of iA and iB.
- Observe how xResult changes based on the comparison.
Operator Precedence in CODESYS
When writing expressions, operator precedence determines the execution order. The following rules apply:
- Parentheses () – Processed first.
- Exponentiation XPT – Next in priority.
- Negation (NOT) – Evaluated before arithmetic operations.
- Multiplication (*), Division (/) – Higher precedence than addition and subtraction.
- Addition (+), Subtraction (-) – Processed next.
- Comparison Operators (>, <, =, <>) – Evaluated before logical operators.
- Logical AND (AND) – Evaluated before OR.
- Logical OR (OR) – Lowest priority.
Example: Evaluating Operator Precedence
xResult := (iA + iB) > 15 AND NOT xButton1;
- iA + iB is calculated first.
- The result is compared to 15.
- NOT xButton1 is applied.
- AND operation is processed last.
Practical Assignment: Ventilator Control Program
To reinforce your understanding of operators, create a ventilator control program using comparison and logical operators.
Requirements:
- Inputs:
- xStartButton: Starts the ventilator.
- rTemperature: Reads the ambient temperature.
- xStopButton: Stops the ventilator.
- Outputs:
- xVentilator: Activates when conditions are met.
Solution:
VAR
xStartButton : BOOL;
xStopButton : BOOL;
rTemperature : REAL;
xVentilator : BOOL;
END_VAR
xVentilator := (xStartButton AND (rTemperature > 25.0)) OR (NOT xStopButton);
Expected Behavior:
- The ventilator turns ON when:
- The start button is pressed AND temperature exceeds 25°C.
- OR if the stop button is NOT pressed.
- The ventilator turns OFF if conditions are not met.
Testing the Program:
- Run the CODESYS simulator.
- Adjust rTemperature values.
- Press and release xStartButton and xStopButton.
- Observe when the ventilator activates.
Conclusion
Operators in CODESYS provide a powerful way to control automation systems. This guide covered:
- Logical operators (AND, OR, NOT, XOR) and their applications.
- Comparison operators (>, <, =, <>) for evaluating conditions.
- Operator precedence and its importance in expression evaluation.
- A real-world programming assignment to practice logical and comparison operators.
By mastering these operators, you can create robust and intelligent control systems in CODESYS. In future projects, combining these operators effectively will be essential for optimizing automation logic.
Free PLC course outline
- Lesson #1: What Is It and Why Should Every PLC Programmer Know It? Part #1
- Lesson #2: How to install Codesys? Part #2
- Lesson #3: Write your first program in Codesys: Structured Text – Part #3
- Lesson #4: How to Create a Codesys Visualization in an Application? – Part #4
- Lesson #5: Introduction to Variables in CODESYS – Part #5
- Lesson #6: Data Structures in CODESYS: Practical Use of Arrays and Structures – Part #6
- Lesson #7: Advanced Data Types. Enumerations and Local vs. Global Variables – Part #7
- Lesson #8: Operators – Introduction and Practical Applications – Part #8
- Lesson #9: Program Flow Control: IF, CASE, and Loops – Part #9