Free PLC course outline
- Lesson #1: What Is It and Why Should Every PLC Programmer Know It? Part #1
- Lesson #2: How to install Codesys? Part #2
- Lesson #3: Write your first program in Codesys: Structured Text – Part #3
- Lesson #4: How to Create a Codesys Visualization in an Application? – Part #4
- Lesson #5: Introduction to Variables in CODESYS – Part #5
- Lesson #6: Data Structures in CODESYS: Practical Use of Arrays and Structures – Part #6
- Lesson #7: Advanced Data Types. Enumerations and Local vs. Global Variables – Part #7
- Lesson #8: Operators – Introduction and Practical Applications – Part #8
- Lesson #9: Program Flow Control: IF, CASE, and Loops – Part #9
In PLC programming, understanding how to use enumerations (ENUM) and the distinction between local and global variables is crucial for writing efficient and structured code. This section explores the use of enumerations in CODESYS, how to declare them, and their advantages in programming projects.
Enumerations in CODESYS data
An enumeration (ENUM) is a user-defined data type that consists of a set of named constants. Enumerations improve code readability, make the program more maintainable, and reduce the likelihood of errors caused by using arbitrary numbers. Instead of using standard integer values that might be confusing, enumerations provide a human-readable way to represent predefined states or options.
Enumerations are especially useful in PLC programming when dealing with state machines, control sequences, or configuration settings, where only a limited set of possible values should be used. By defining an enumeration, we ensure that variables take on only valid values, reducing the risk of unexpected behavior in the program.
Declaring an Enumeration in CODESYS
To define an enumeration, use the following syntax:
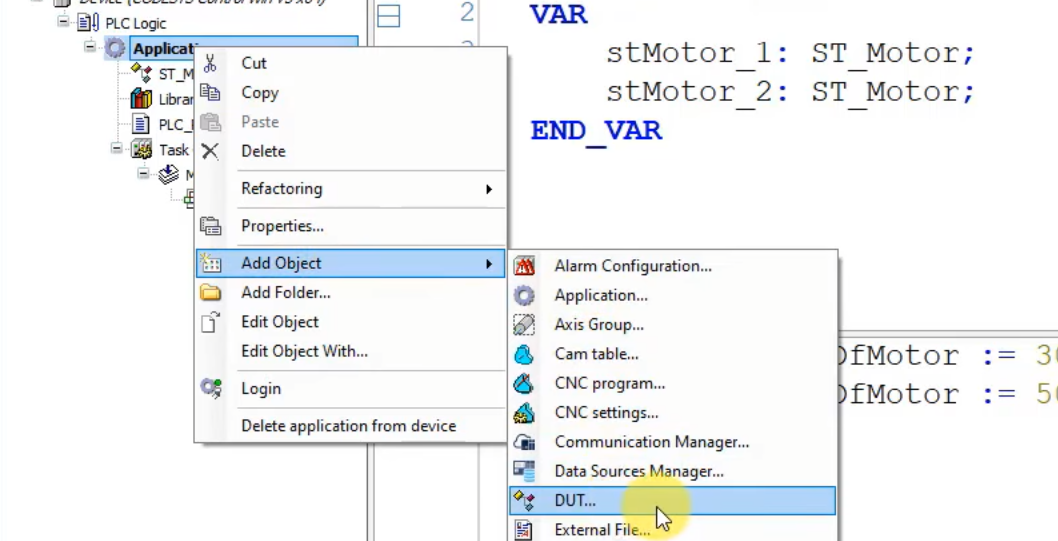
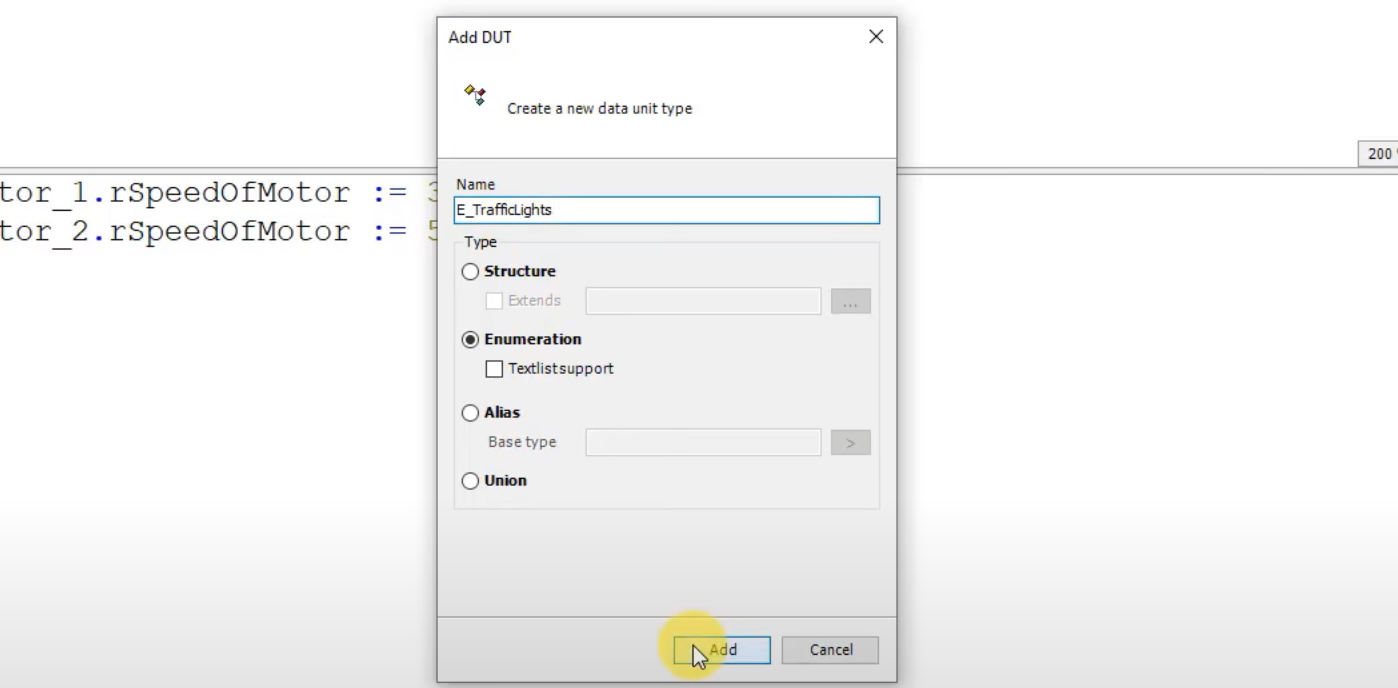
TYPE E_TrafficLights:
(Yellow := 0, Green := 1, Red := 2);
END_TYPE
Here, we define an enumeration named E_TrafficLight, which includes three states: Yellow, Green, and Red. Each state is assigned a numeric value for internal representation. These values serve as constant references within the program and help ensure consistency when referring to specific conditions.
Declaring and Using an Enumeration Variable
Once the enumeration is created, we can declare a variable using this type:
VAR
eTrafficLights : E_TrafficLights;
iColor : INT;
END_VAR
This allows us to store and manipulate enumeration values while keeping the code readable and structured.
Assigning and Using Enumeration Values in Programs
We can assign values to an enumeration variable as follows:
iColor := eTrafficLights;
This stores the numeric equivalent of eTrafficLights in the integer variable iColor. This is particularly useful when working with hardware interfaces or functions that expect numerical values.
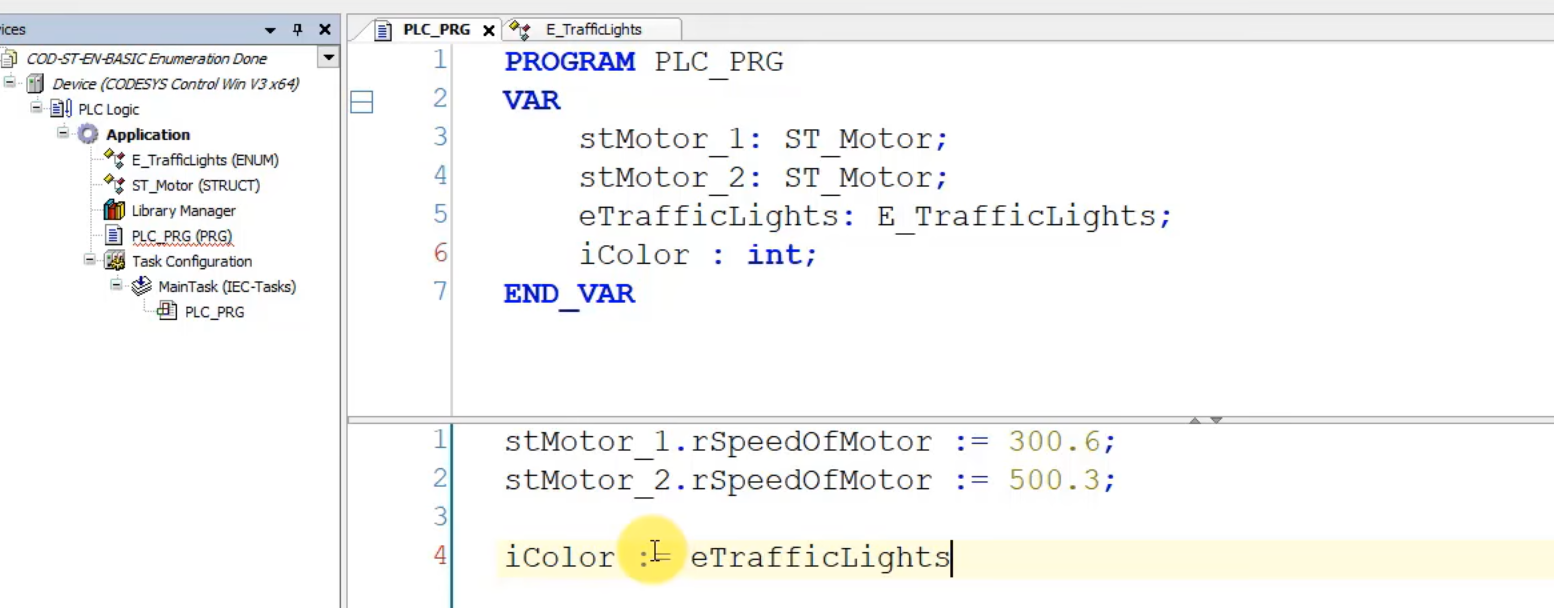
Using Enumeration in a PLC Program
Enumerations are useful in state management and structured programming. Below is an example of how enumerations help manage traffic light states:
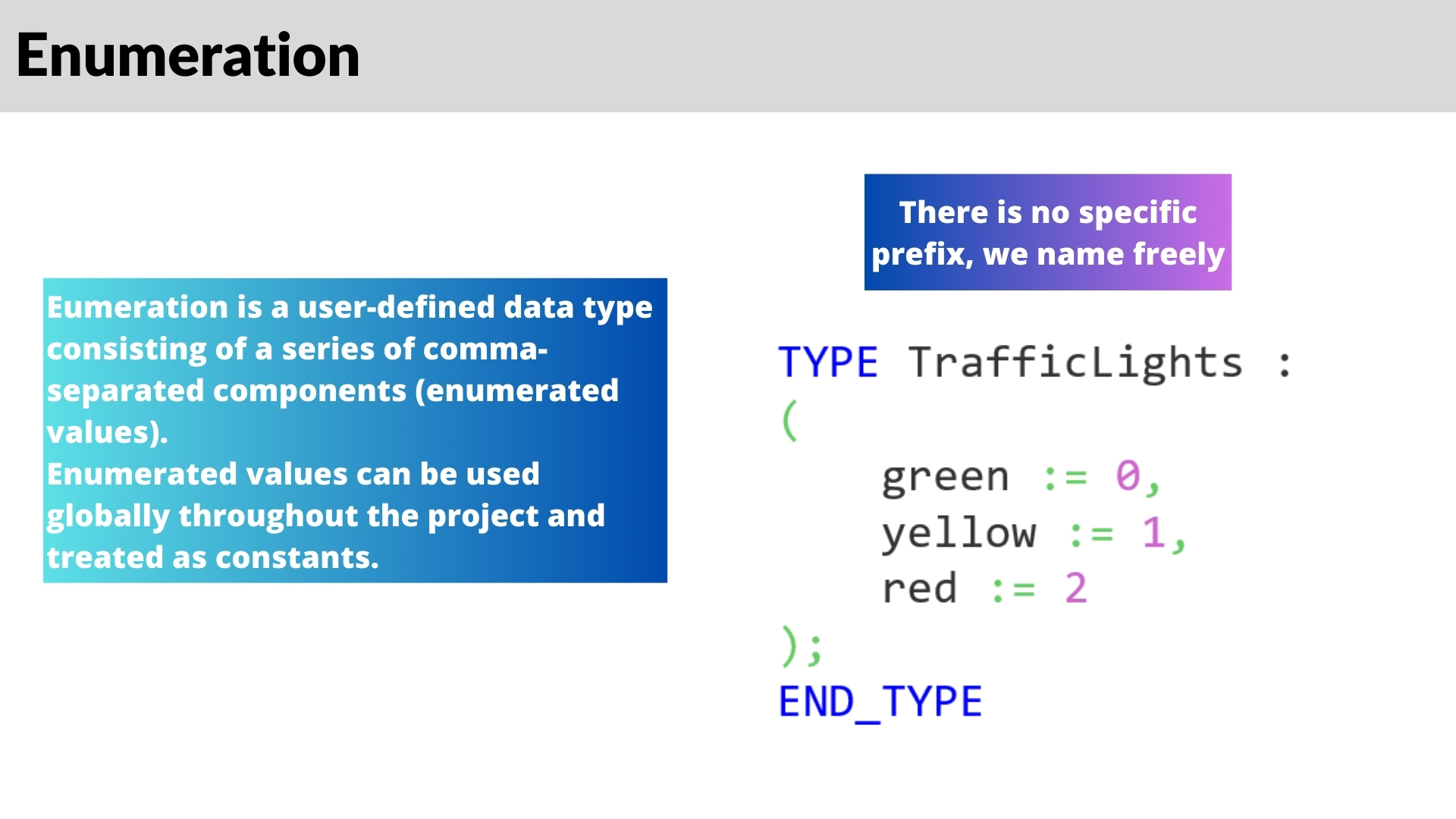
Using enumerations in this way ensures that all possible values are predefined and handled in a controlled manner. This eliminates the need to compare raw integer values, making the program easier to debug and maintain.
Assigning Enumeration Values from a Drop-Down List
CODESYS provides a useful feature that allows selection of enumeration values from a drop-down list in the interface. This helps developers choose from valid options without manually entering values, reducing errors.
For example, when assigning a new value to an enumeration variable in the CODESYS editor, a drop-down list appears, displaying the available options (Yellow, Green, Red). This feature speeds up programming and prevents accidental assignments of invalid values.
Real-World Applications of Enumerations
Enumerations are widely used in industrial automation, particularly in the following scenarios:
- Machine State Control: Representing states such as Idle, Running, Error, and Maintenance.
- Mode Selection: Configuring devices to run in different modes, such as Manual, Automatic, or Semi-Automatic.
- Alarm and Fault Handling: Defining error codes and alarm levels, e.g., LowAlarm, HighAlarm, CriticalAlarm.
- Traffic Light Control: Managing the states of traffic signals, as shown in our previous example.
By using enumerations instead of raw numbers, the readability and reliability of the code are greatly improved, making the system easier to maintain and scale.
Advantages of Using Enumerations
- Improved Readability: Instead of using arbitrary numbers, names are assigned to specific values, making the program easier to understand.
- Error Prevention: Prevents the use of invalid values, ensuring that only predefined states are used.
- Simplified Debugging: Makes it easier to identify what each value represents, reducing confusion.
- Consistency Across Projects: Ensures uniform naming conventions for constants, making the project more structured.
- Faster Development: Reduces the need for excessive comments and documentation, as the enumeration names describe their function directly.
Understanding Local and Global Variables in CODESYS
In PLC programming, understanding the distinction between local and global variables is crucial for creating structured, efficient, and maintainable code. This section will explore their differences, best practices, and a practical assignment to reinforce the concepts.
What Are Local Variables?
Local variables are declared within a specific program organization unit (POU), such as a function block, function, or program. These variables are only accessible within that unit and cannot be referenced elsewhere.
Example of Local Variable Declaration
PROGRAM Test_PRG
VAR
iLocalVar : INT;
END_VAR
Here, I_LocalVar is a local integer variable that can only be accessed within the Test_PRG program.
What Are Global Variables?
Global variables, on the other hand, can be accessed from any part of the project. They are typically declared in a Global Variable List (GVL) and used for data exchange between different POUs.
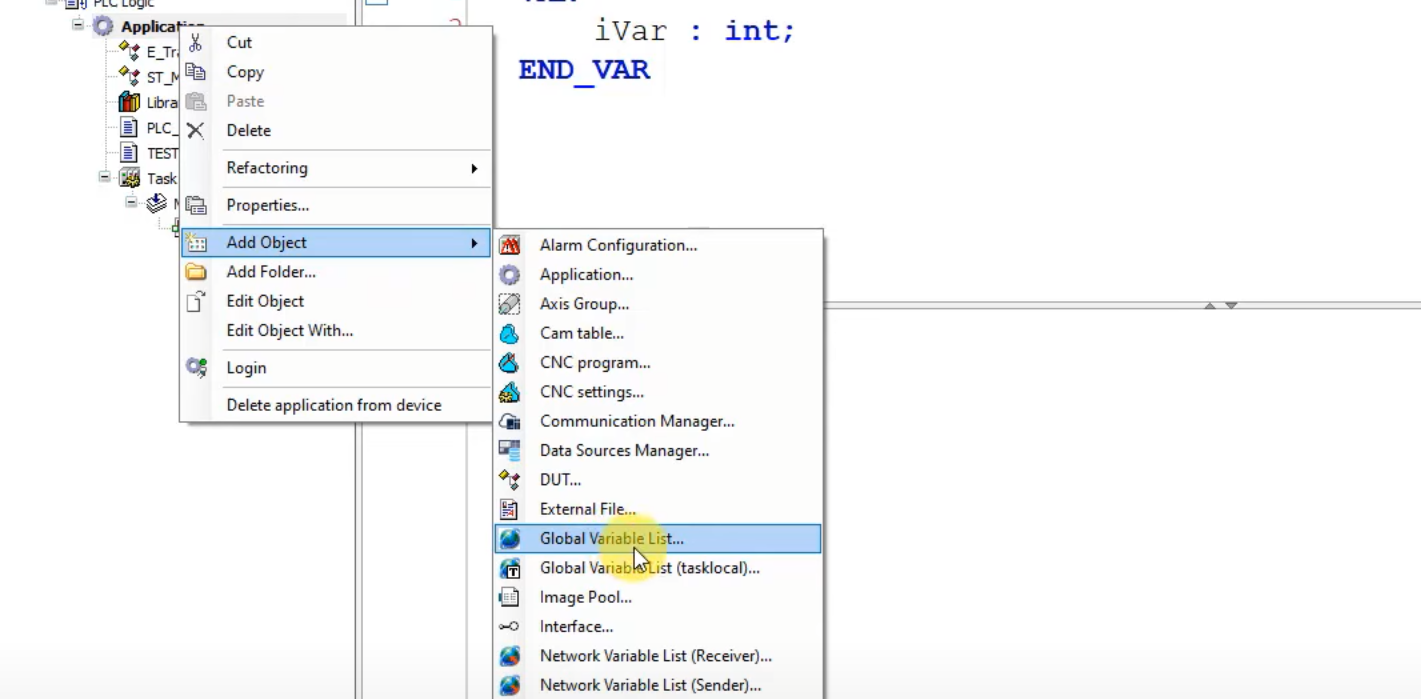
Example of Global Variable Declaration
VAR_GLOBAL
iGlobalVar : INT;
xLamp : BOOL;
END_VAR
Global variables provide convenient data sharing but must be used carefully to avoid unintended modifications and dependencies.
When to Use Local vs. Global Variables
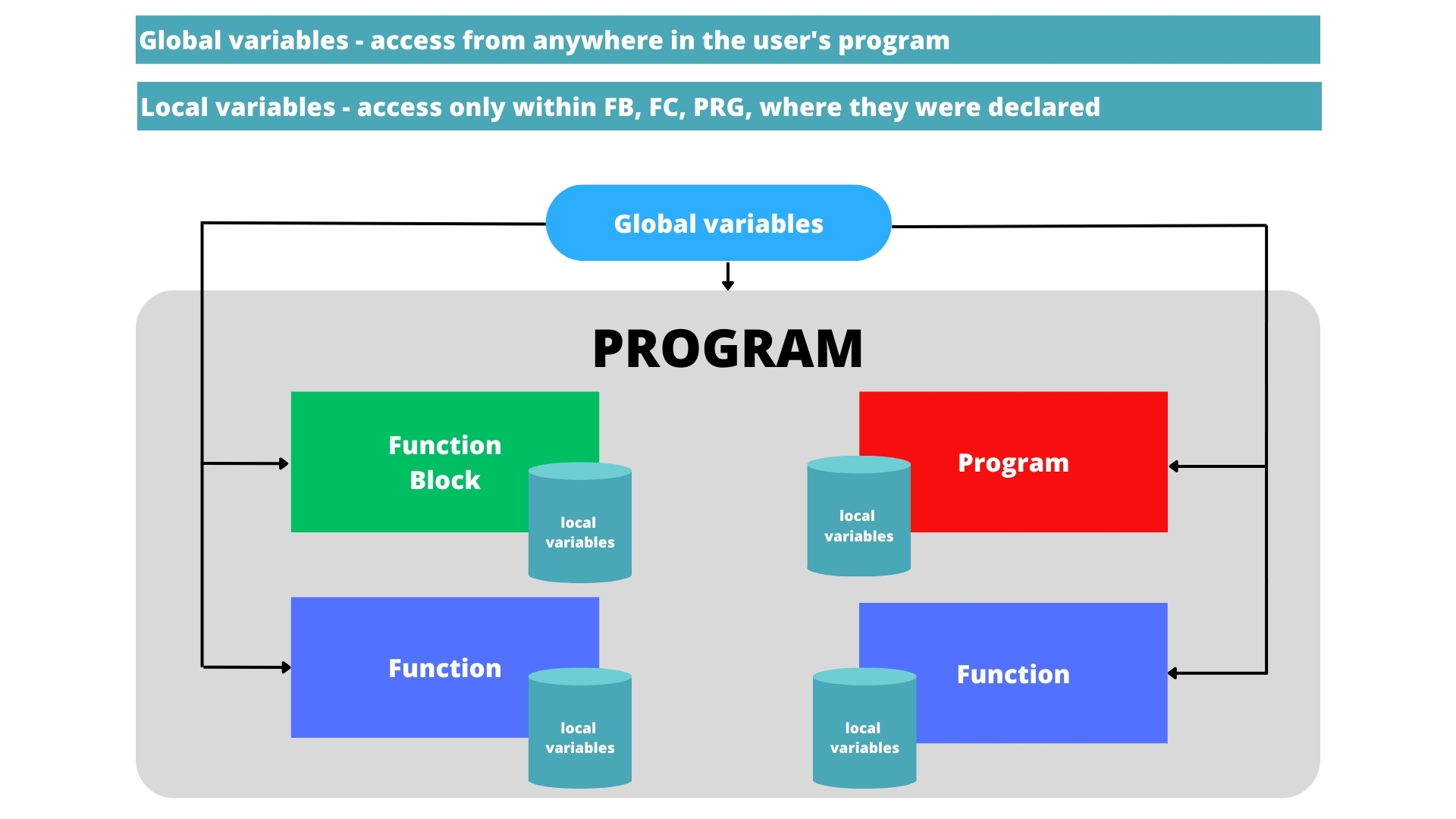
Best Practices for Using Local Variables
- Encapsulation: Keep variables within their relevant program blocks to prevent unintended modifications.
- Readability: Easier to track variable usage and prevent conflicts.
- Memory Efficiency: Local variables are only active when their function or program is executed, minimizing memory usage.
When to Use Global Variables
- Data Exchange Between POUs: When different programs need to share data, global variables help facilitate communication.
- Configuration Parameters: Global variables are useful for storing settings or parameters that need to persist throughout the program execution.
- I/O Mapping: Often used to define variables linked to physical inputs and outputs.
Practical Assignment: Working with Variables
To reinforce the concepts of local and global variables, follow the instructions below and complete the programming challenge.
Step 1: Declare Variables
Create a new CODESYS project and declare the following variables:
Local Variables
VAR
iDate : INT;
wDate : WORD;
uiYear : UINT;
sDay : STRING;
rTemperature : REAL;
END_VAR
Each variable represents a different data type:
- iDate: Stores the day and month as an integer.
- wDate: Stores the date in binary format.
- uiYear: Stores the year in octal format.
- sDay: Stores the day of the week as a string.
- rTemperature: Stores the temperature as a floating-point number.
Step 2: Assign Values to Variables
Use today’s date and assign values in different numeric formats:
// Date: 13/09/2024, Wednesday
iDate := 1309; // 13th of September
wDate := 2#101000101; // Binary representation
uiYear := 8#2023; // Octal notation
sDay := 'Wednesday'; // String variable
rTemperature := 24.6; // Actual outside temperature
This exercise helps reinforce data representation in different numeric systems.
Step 3: Working with Arrays
Next, declare an array with three elements to store the date components: Day, Month, Year
Now assign values to the array:
aArray[0] := 13; // Day
aArray[1] := 9; // Month
aArray[2] := 2023; // Year
Step 4: Perform Mathematical Operations on Arrays
Using these date values, perform a simple calculation:
iResult := aArray[0] + aArray[2] - aArray[1];
This sums the day and year and subtracts the month, showcasing basic arithmetic operations using arrays.
Step 5: Testing the Program in CODESYS
- Compile the program and simulate the execution.
- Ensure that all variables are correctly initialized and displayed.
- Modify the values and observe how changes affect the calculations.
Summary
In PLC programming, understanding enumerations (ENUM) and the distinction between local and global variables is essential for writing efficient and structured code. Enumerations in CODESYS enhance code readability, minimize errors, and facilitate state management in automation systems. They are particularly useful in applications such as machine state control, mode selection, and alarm handling.
Additionally, the proper use of local and global variables impacts code structure and security. Local variables improve encapsulation and memory efficiency, while global variables enable seamless data exchange between different program units. The article also provides practical exercises on variable declarations, assignments, arrays, and mathematical operations.
By mastering these concepts in CODESYS, developers can create more organized, scalable, and reliable automation programs, ensuring better system maintainability and performance.
Free PLC course outline
- Lesson #1: What Is It and Why Should Every PLC Programmer Know It? Part #1
- Lesson #2: How to install Codesys? Part #2
- Lesson #3: Write your first program in Codesys: Structured Text – Part #3
- Lesson #4: How to Create a Codesys Visualization in an Application? – Part #4
- Lesson #5: Introduction to Variables in CODESYS – Part #5
- Lesson #6: Data Structures in CODESYS: Practical Use of Arrays and Structures – Part #6
- Lesson #7: Advanced Data Types. Enumerations and Local vs. Global Variables – Part #7
- Lesson #8: Operators – Introduction and Practical Applications – Part #8
- Lesson #9: Program Flow Control: IF, CASE, and Loops – Part #9