Free PLC course outline
- Lesson #1: What Is It and Why Should Every PLC Programmer Know It? Part #1
- Lesson #2: How to install Codesys? Part #2
- Lesson #3: Write your first program in Codesys: Structured Text – Part #3
- Lesson #4: How to Create a Codesys Visualization in an Application? – Part #4
- Lesson #5: Introduction to Variables in CODESYS – Part #5
- Lesson #6: Data Structures in CODESYS: Practical Use of Arrays and Structures – Part #6
In PLC programming, working with more complex data structures is essential for handling numerical computations, collections of data, and structured information. Efficient use of these structures improves program readability, optimizes memory usage, and simplifies data management. This section explores floating-point numbers (REAL), arrays (ARRAYS), and structures (STRUCTURE) in CODESYS, demonstrating their significance and practical usage.
Floating-Point Numbers (REAL) in CODESYS
Floating-point numbers are used to store decimal values, which are necessary for representing physical quantities such as temperature, pressure, or flow rates. Many real-world measurements require precision beyond whole numbers, making floating-point data types indispensable in industrial automation.
In CODESYS, floating-point numbers are categorized into two main types:
- REAL: Occupies 32 bits, offering moderate precision and range.
- LONG REAL: Occupies 64 bits, providing an extended range and higher precision.
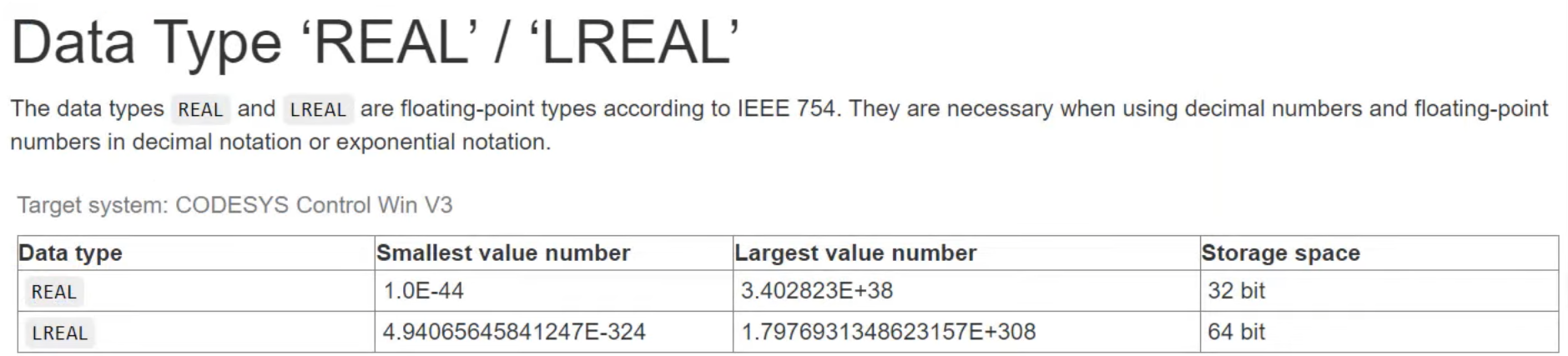
Advantages of Floating-Point Numbers
- Allow precise representation of decimal values.
- Essential for analog sensor readings and complex calculations.
- Enable fractional arithmetic in control systems, avoiding integer rounding errors.
To explore these data types further, you can access the CODESYS online help under Data Types -> REAL and LONG REAL.
Declaring a Floating-Point Variable in CODESYS
- Open CODESYS and navigate to PLC_PRG.
- Declare a floating-point variable as follows: rResult : REAL := 1,345678;
- Click Enter to confirm the declaration.
- Log in to the PLC simulator and start execution.
- Observe the approximation of the displayed value.
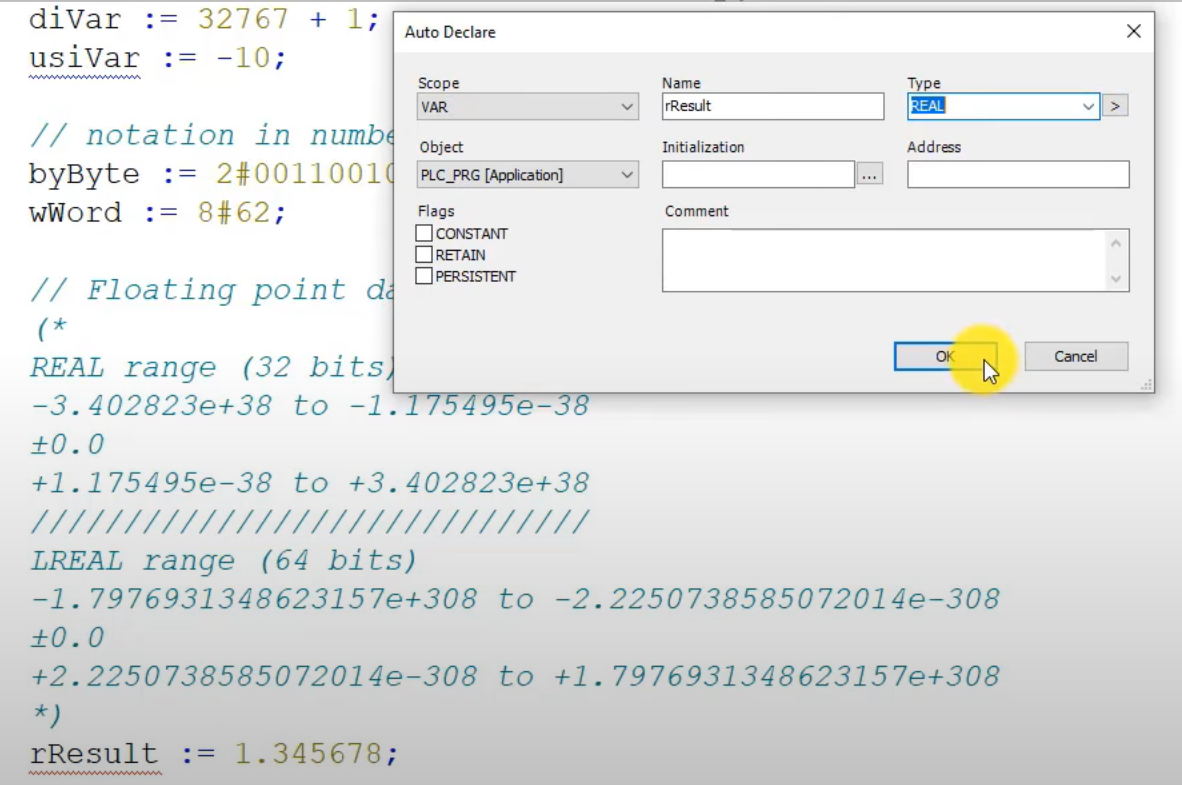

Since floating-point variables are stored in binary, some decimal values cannot be represented precisely. When hovering over the value, CODESYS displays the full stored value, providing a clearer picture of the internal representation.
Application: Floating-point numbers are frequently used in automation projects requiring precise numerical calculations, such as PID control algorithms, temperature monitoring, and pressure regulation.
Arrays in CODESYS
An array is a structured collection of multiple elements of the same data type, allowing efficient organization and manipulation of related values. Arrays in CODESYS provide a structured way to store multiple values without the need to declare separate variables for each.
Benefits of Using Arrays
- Reduce the number of declared variables.
- Enable easy indexing and iteration through multiple values.
- Improve data structure organization in control applications.
Declaring an Array in CODESYS
- Open CODESYS and go to PLC_PRG.
- Use the following syntax to declare an array: ARR_Data : ARRAY[0..10] OF INT;
- This statement creates an integer array with 11 elements (from index 0 to 10).
- To initialize an array with values, use the Auto Declare feature:
- Open Edit → Auto Declare.
- Enter the array name and set the initialization values.
- Click OK to confirm.
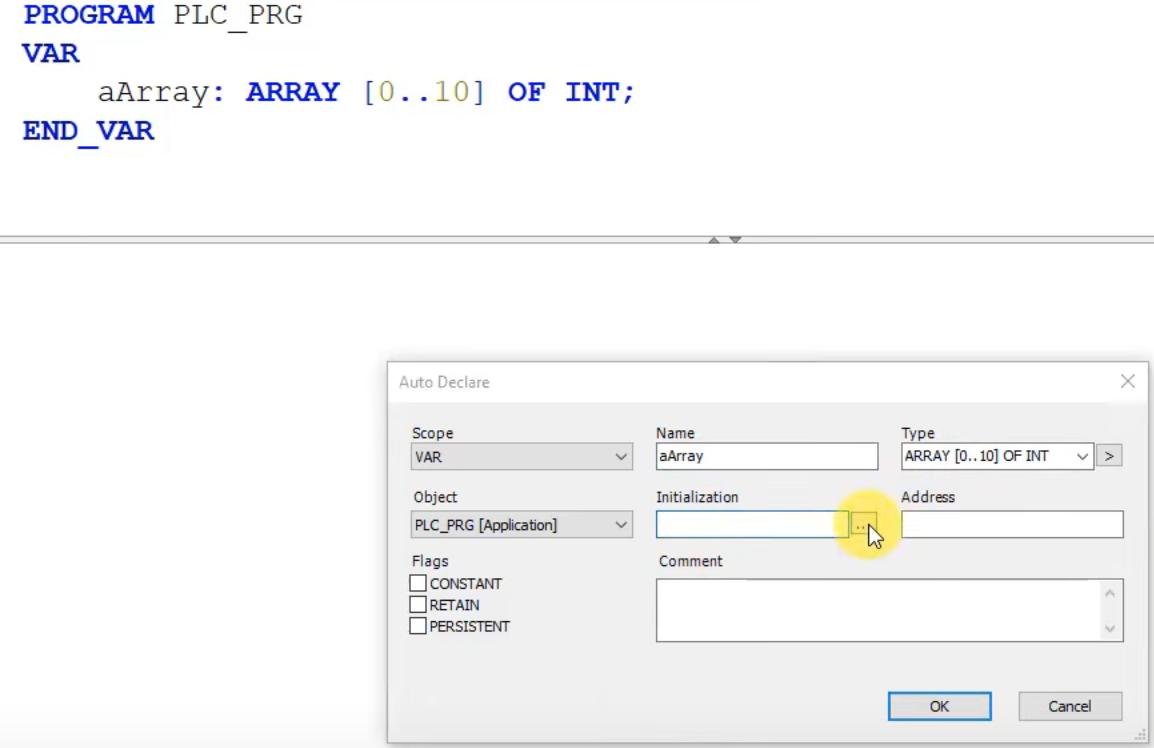
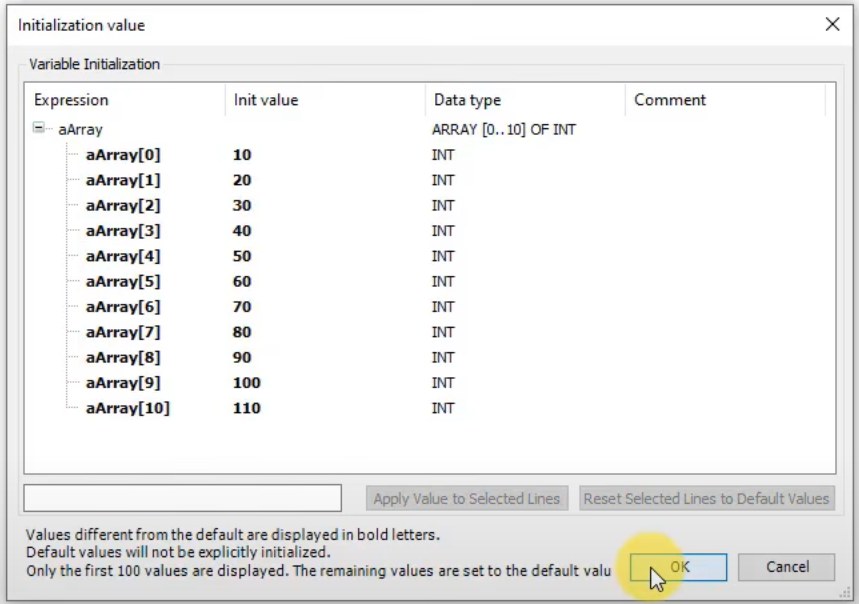
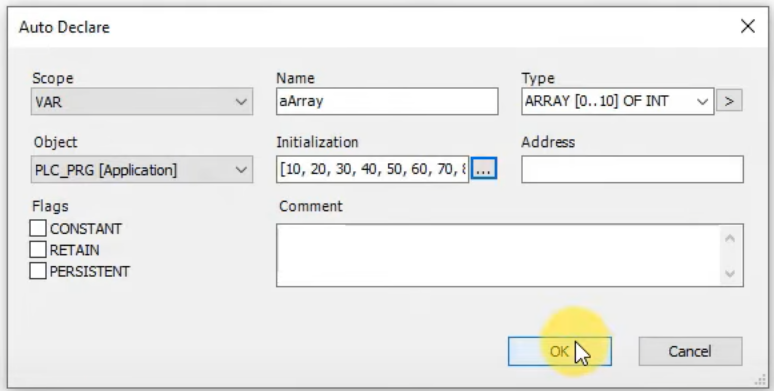
Accessing Array Elements
To retrieve or modify an array element:
iVar := aArray[3];
This assigns the value stored at index 3 of aArray to the integer variable iVar.

Testing an Array in CODESYS
- Log in to the PLC simulator.
- Check the Simulation option.
- Run the program and observe the values.
- Modify specific array elements and verify changes in the runtime environment.
Arrays are particularly useful for sensor data storage, buffer implementations, iterative computations, and batch processing in PLC applications.
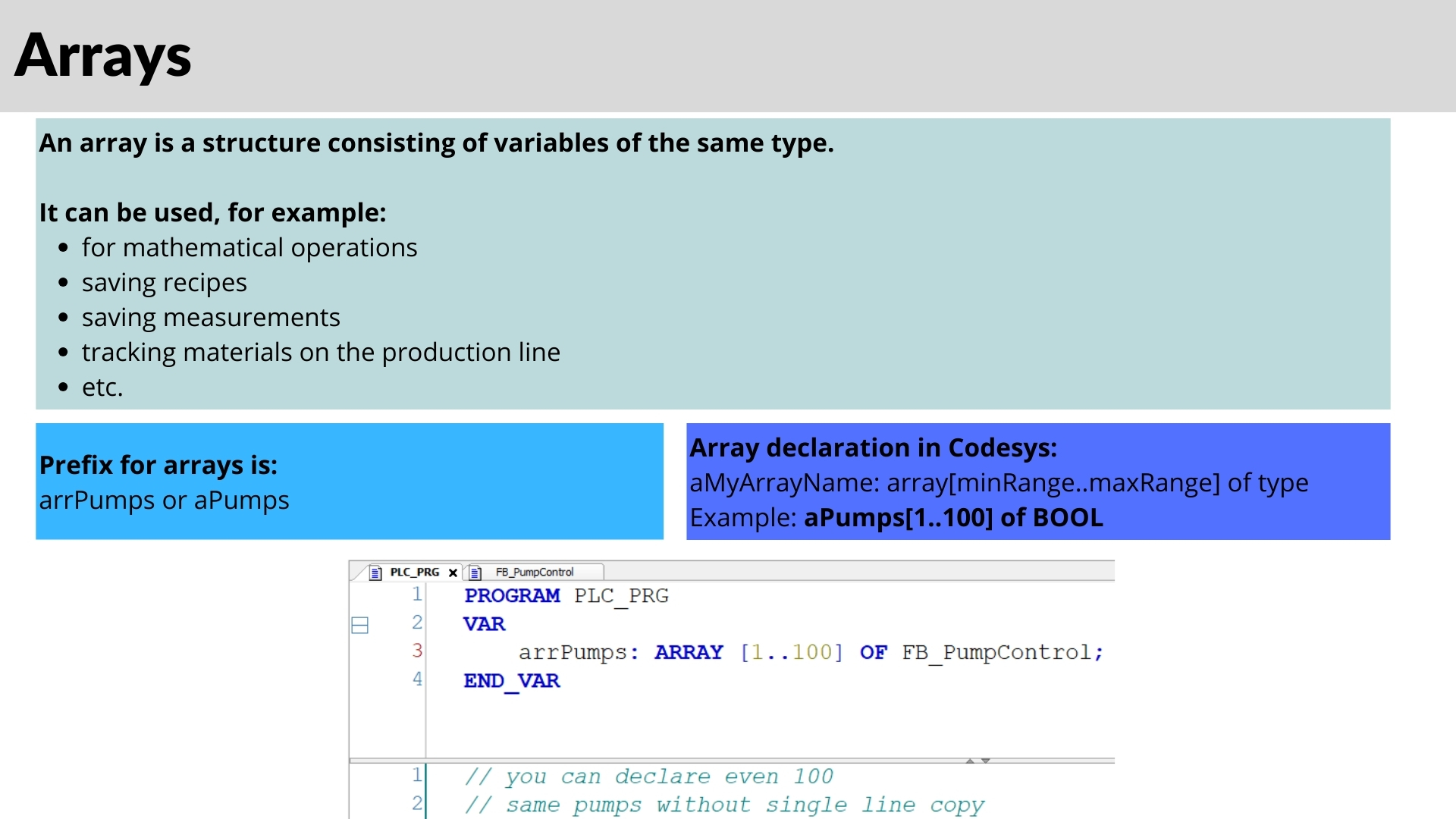
Declaring and Using Structures in a Program
Structures in CODESYS allow developers to define a custom data type that groups multiple related variables together. This makes the code more readable, scalable, and efficient when working on large automation projects.
Defining a Custom Structure
To define a new structure type, follow these steps:
TYPE ST_Motor:
STRUCT
rSpeed: REAL;
rCurrent: REAL;
rVoltage: REAL;
iTemperature: INT;
xThermalSensor: BOOL;
END_STRUCT;
END_TYPE
This structure represents a motor, storing its speed, current, and operational state.
Declaring Structured Variables
After defining the structure, we can declare variables of this type:
PROGRAM PLC_PRG
VAR
stMotor_1 : ST_Motor;
stMotor_2 : ST_Motor;
END_VAR
Each motor now has its own independent variables for speed, current, and operational status.
Assigning Values to Structure Members
To assign values to the members of the structure:
stMotor_1.rSpeed := 300.6;
stMotor_2.rSpeed := 500.3;
This method ensures clear and organized data management in PLC applications.

Using Structures in Large Projects
Structures are especially useful in large-scale automation projects where multiple instances of similar components exist. For example, instead of creating separate variables for each motor, you can use arrays of structures. This simplifies managing multiple motors, making the program modular and scalable.
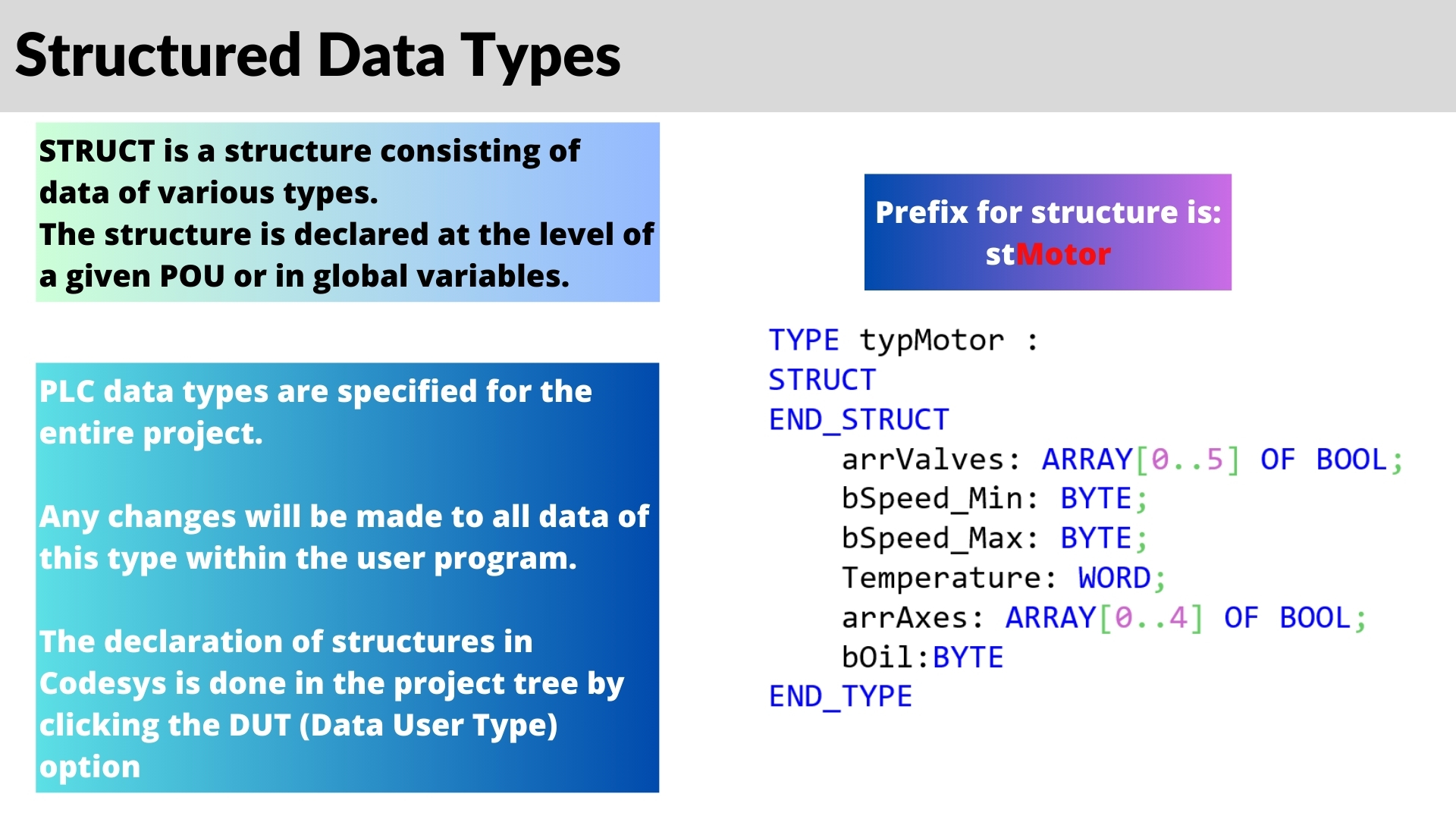
Difference Between Structures and Local Variables
- Structures are defined globally and apply to the entire project.
- Local variables exist only within the function or program where they are declared.
- Structures use a capitalized ST prefix in the declaration, while variable instances follow lowercase notation.
Best Practices for Using Structures in CODESYS
1. Use Structures for Grouping Related Data
- Structures improve modularity by grouping similar variables together.
- Reduces the number of individual variable declarations.
- Enhances code readability and maintainability.
2. Use Arrays of Structures for Large-Scale Applications
- Arrays of structures simplify handling multiple similar components (e.g., motors, sensors).
- Allows easy iteration over multiple objects with loops.
3. Define Structures Globally for Reusability
- Helps maintain consistency across the entire project.
- Reduces redundancy and enhances reusability.
4. Follow a Consistent Naming Convention
Ensure meaningful and intuitive names for better readability.
Use st as a prefix for structures (e.g., stMotor).
Summary:
This article explores the use of real arrays and structures in CODESYS, demonstrating how to declare, initialize, and effectively use them in PLC programming. Through practical examples, the author explains how these data structures can enhance code organization and improve control logic implementation. Readers will gain insights into handling structured data efficiently within CODESYS applications.
Free PLC course outline
- Lesson #1: What Is It and Why Should Every PLC Programmer Know It? Part #1
- Lesson #2: How to install Codesys? Part #2
- Lesson #3: Write your first program in Codesys: Structured Text – Part #3
- Lesson #4: How to Create a Codesys Visualization in an Application? – Part #4
- Lesson #5: Introduction to Variables in CODESYS – Part #5
- Lesson #6: Data Structures in CODESYS: Practical Use of Arrays and Structures – Part #6