Free PLC course outline
- Lesson #1: What Is It and Why Should Every PLC Programmer Know It? Part #1
- Lesson #2: How to install Codesys? Part #2
- Lesson #3: Write your first program in Codesys: Structured Text – Part #3
- Lesson #4: How to Create a Codesys Visualization in an Application? – Part #4
- Lesson #5: Introduction to Variables in CODESYS – Part #5
- Lesson #6: Data Structures in CODESYS: Practical Use of Arrays and Structures – Part #6
In PLC programming, especially in CODESYS, understanding how data is stored and organized in the controller’s memory is crucial. Proper variable management allows optimal utilization of hardware resources and ensures the correct operation of the control program. In this article, we will discuss basic memory units, variable types, and constants used in PLC applications.
Memory Units in CODESYS
CODESYS operates on memory divided into units of different sizes:
- Bit – the smallest memory unit that can store a logical value of 1 (TRUE) or 0 (FALSE). It is used for storing simple digital signals, such as switch states.
- Byte – consists of 8 bits, with each bit capable of storing an individual logical value. It is used for storing small numerical values or ASCII characters.
- Word – consists of 16 bits (2 bytes). It is commonly used in PLCs to store integer values and memory addresses.
- Double Word (DWORD) – consists of 32 bits (4 bytes, or two words). It is used for storing larger values, such as floating-point numbers.
Memory in CODESYS is organized into words of 16-bit size. For more advanced operations, double words (DWORD) are used, allowing the storage of larger numerical values and more complex data structures.
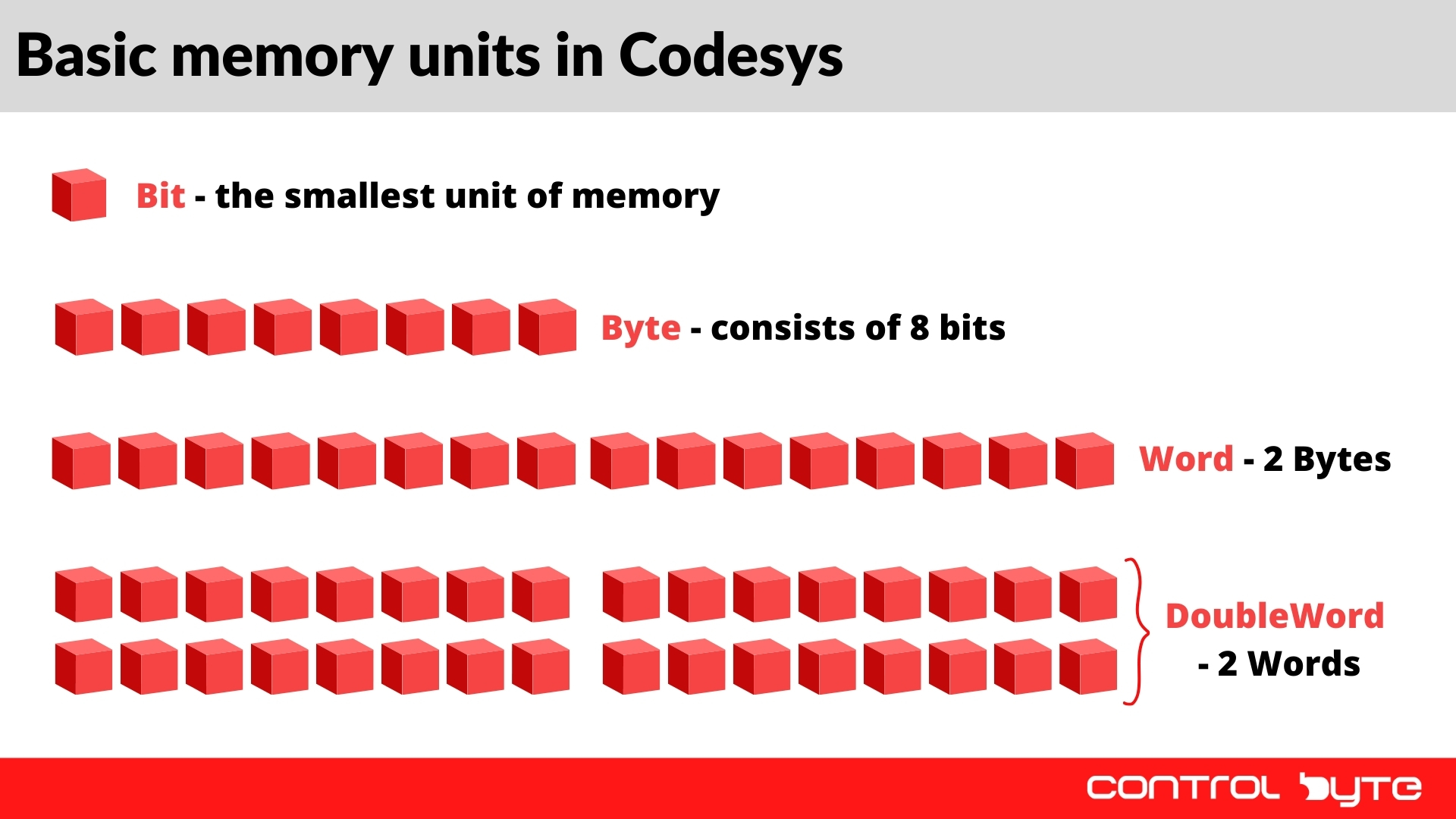
Tip
To efficiently manage memory, it is important to understand its structure and different addressing methods. This helps avoid wasted memory and optimizes resource management in PLC projects. Additionally, in more advanced applications, managing variable addressing effectively prevents overwriting critical data. In CODESYS, memory addresses for variables can be precisely defined, which is crucial when working with physical hardware.
For example, allocating memory for variables thoughtfully can optimize controller performance. Using appropriately typed variables minimizes memory usage and improves the efficiency of the control application.
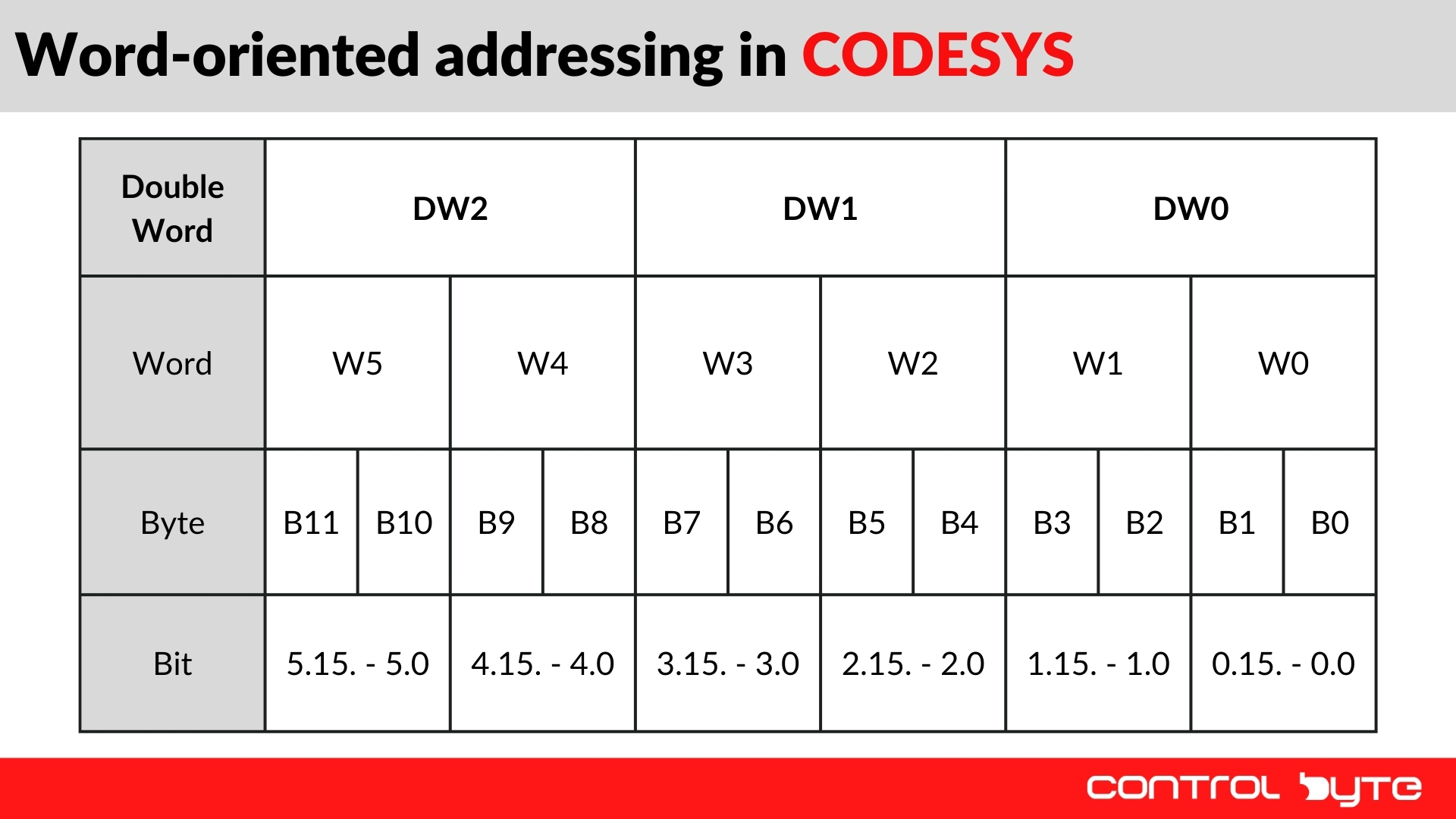
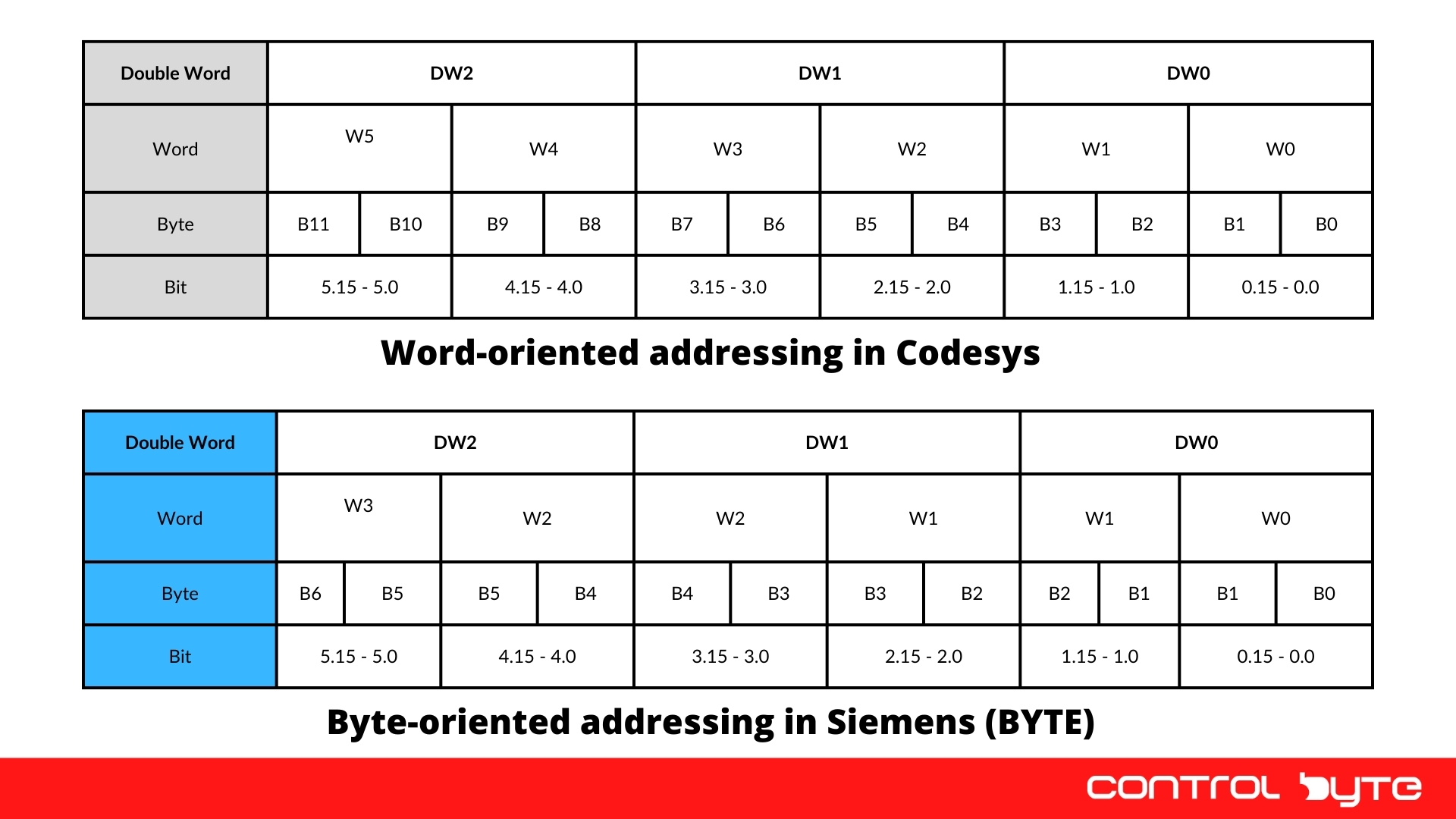
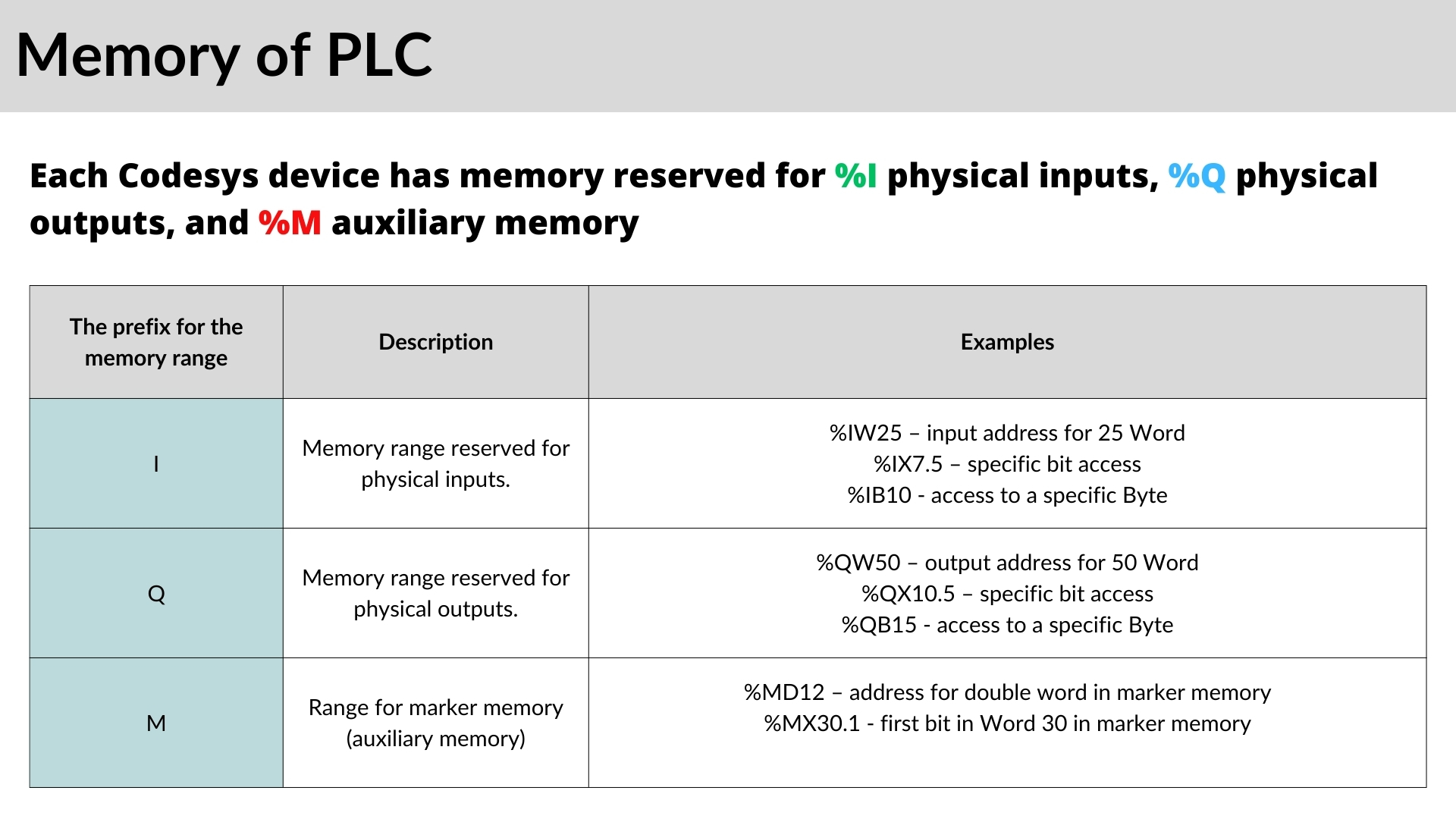
Variables and Constants in CODESYS
Definition of a Variable
In simple terms, a variable is a memory location in the PLC where data is stored. Each variable has the following attributes:
- Data Type – e.g., Boolean, Integer, Real.
- Name – assigned by the programmer, such as xLamp (Boolean) or iTemperature (Integer).
- Memory Address – a unique location where the variable is stored in the PLC memory.
Variables can change values during program execution, making them a fundamental element of process control in PLCs. They allow the storage of calculation results, sensor states, and other essential data required for control applications.
In CODESYS, variables can be declared in different ways depending on their purpose and context. These include local variables (available only within a specific program), global variables (accessible throughout the entire project), and system variables used for communication with external devices.
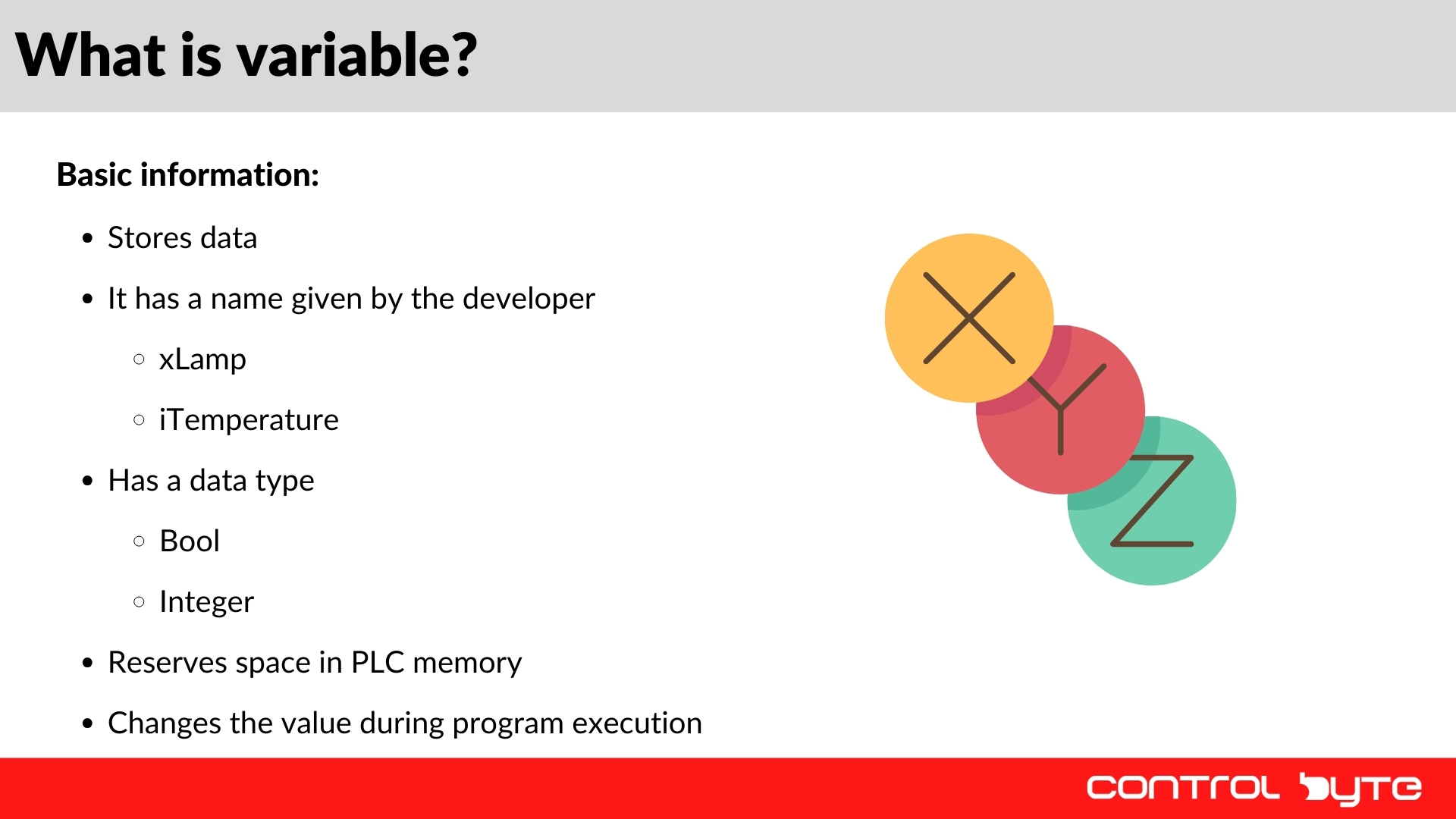
Constants
Constants are values that do not change during program execution. Like variables, they have a data type and a symbolic name. An example of a constant is the number Pi = 3.14, which remains unchanged. Constants are often used to store configuration parameters or reference values that should not be modified by the user.
Declaring constants in the code simplifies project management and enhances readability. Instead of hardcoding values multiple times, constants can be referenced by name, making modifications easier.
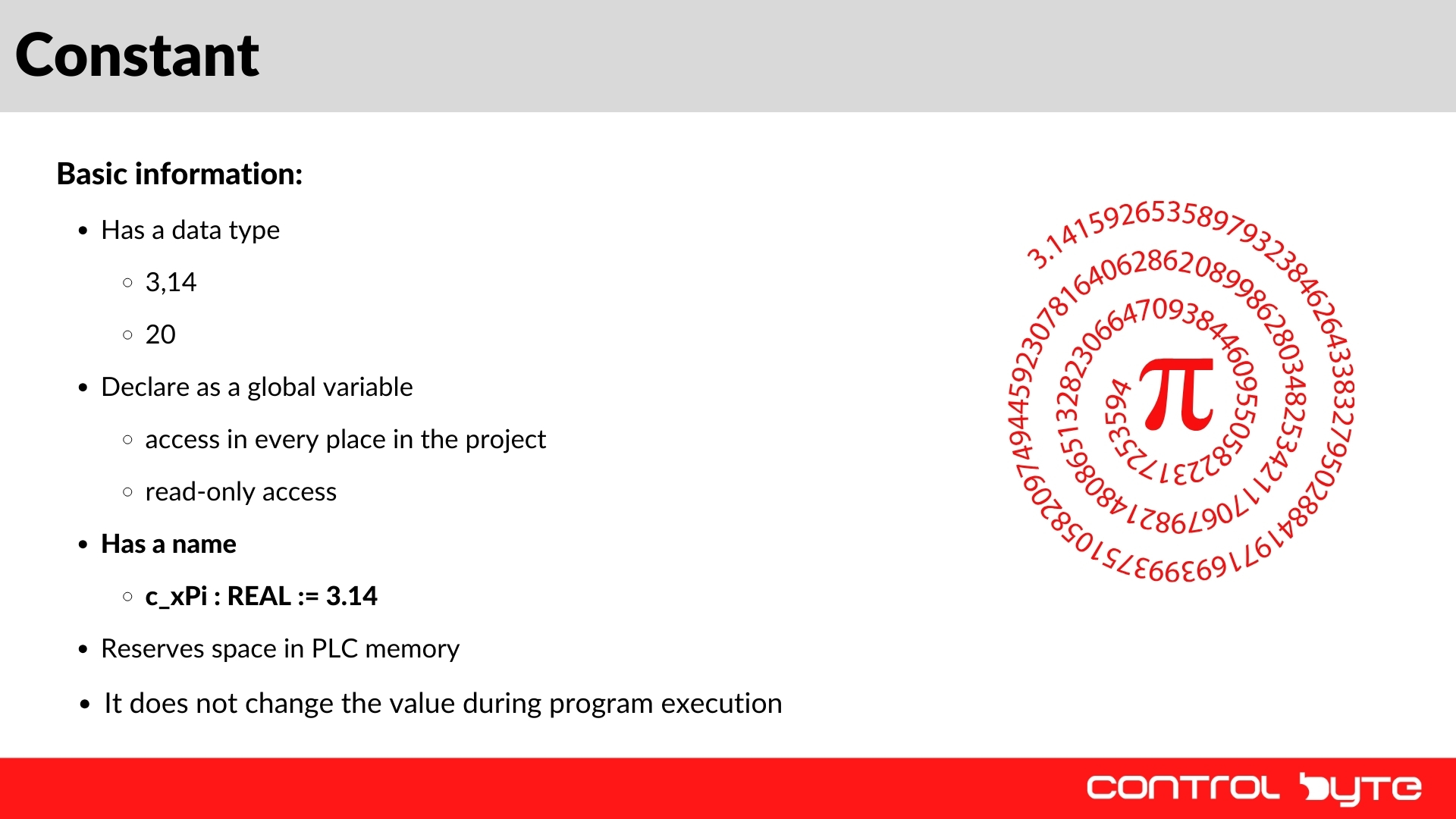
Declaring a Boolean Variable in CODESYS
Step 1: Creating a New Project
- Open CODESYS and create a new project.
- Navigate to PLC_PRG.
Step 2: Adding a Variable
- In the first line, type the variable name using Hungarian notation: xVar := True;
- x denotes a Boolean type.
- Var is the variable name.
- := is the assignment operator in Structured Text (ST).
- TRUE is the initial value.
- Every line in Structured Text must end with a semicolon (;).
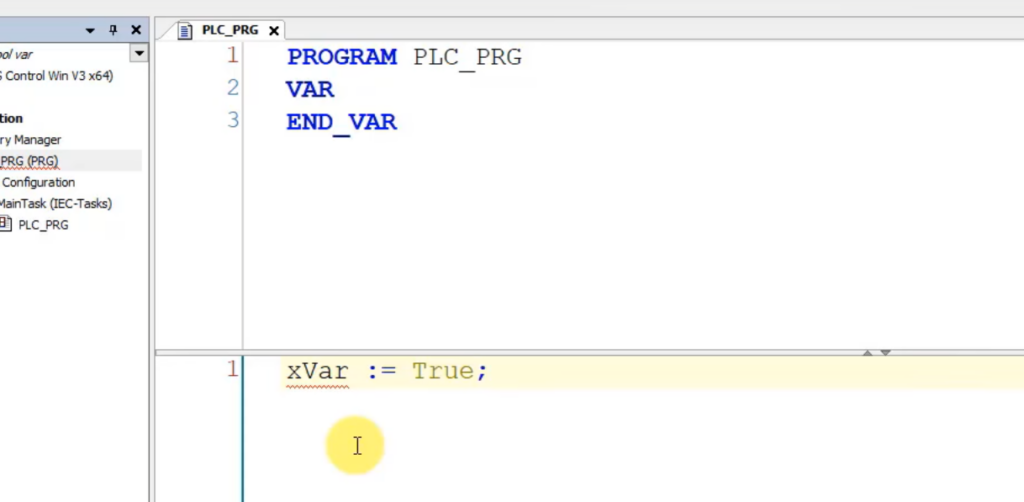
Step 3: Adding a Comment
Adding comments improves code readability:

Step 4: Using the “Auto Declare” Feature
- Press Enter – the “Auto Declare” window appears.
- Fill in the fields:
- Name: xVar
- Data Type: BOOL
- Scope: Local Variable
- Initialization: FALSE
- Click OK to add the variable to the program.
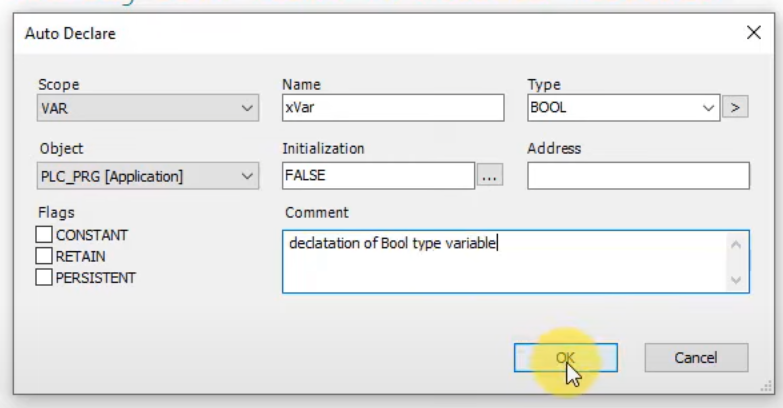
Integer data type
Understanding integer data types in CODESYS is crucial for effective PLC programming. CODESYS provides different types of integers that vary based on their range and whether they include signed or unsigned values. These integer types are essential for handling numerical operations efficiently and avoiding errors related to data overflow.
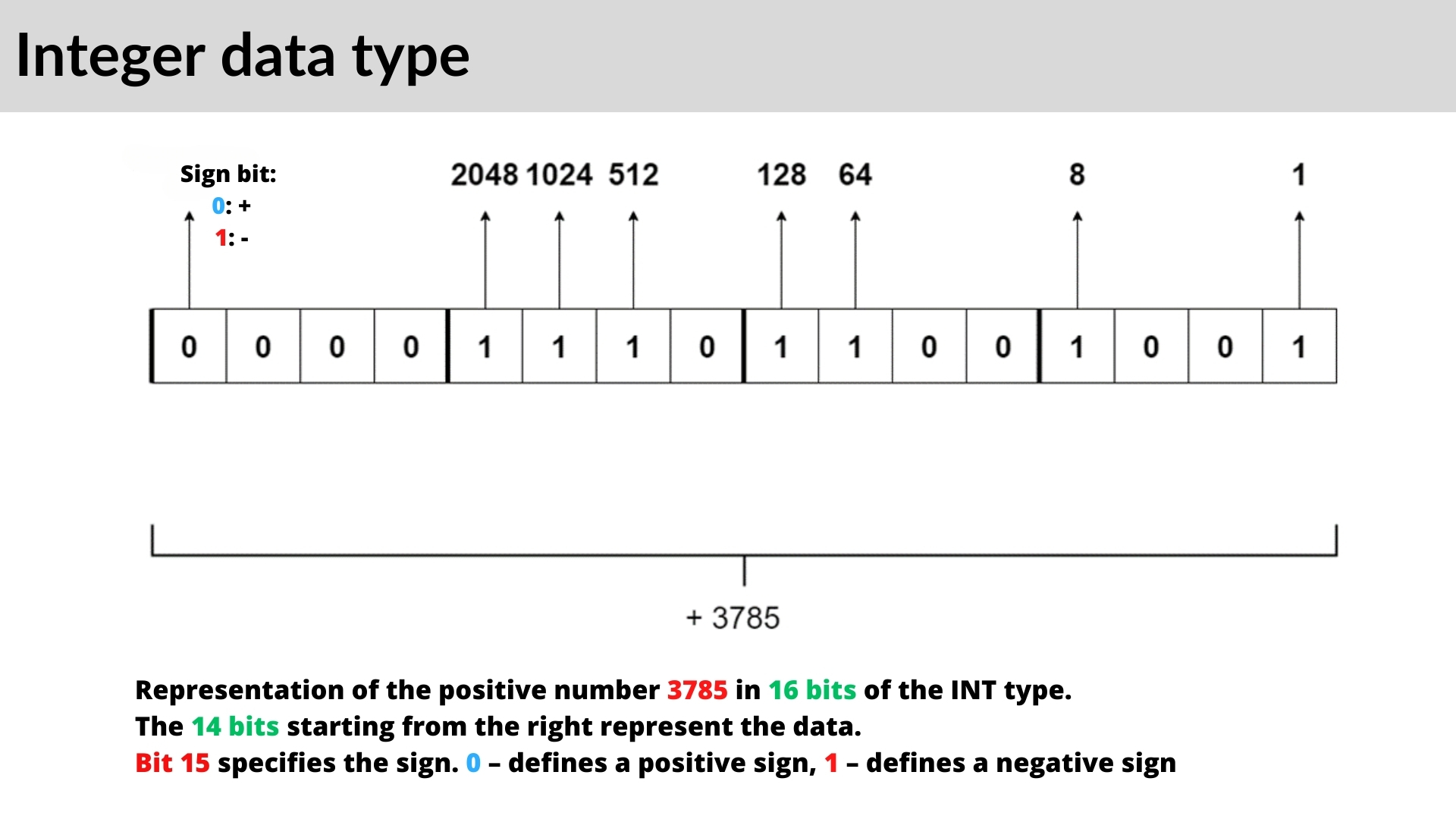
Types of Integer Data Types in CODESYS
CODESYS supports the following integer data types:
- Signed Integer (INT) – A standard integer that includes negative values. The lower limit is -32,768, and the upper limit is 32,767, occupying 16 bits of memory.
- Short Integer (SINT) – A smaller signed integer with a range from -128 to 127, using only 8 bits of memory.
- Double Integer (DINT) – A larger integer with a range of -2,147,483,648 to 2,147,483,647, occupying 32 bits of memory.
- Unsigned Short Integer (USINT) – This integer does not allow negative values, with a range of 0 to 255, using 8 bits.
- Unsigned Integer (UINT) – A 16-bit unsigned integer with a range of 0 to 65,535.
- Unsigned Double Integer (UDINT) – A 32-bit unsigned integer, ranging from 0 to 4,294,967,295.
To find more details about integer types in CODESYS, you can refer to CODESYS Help under Reference -> Programming -> Data Types -> Integer Data Types.
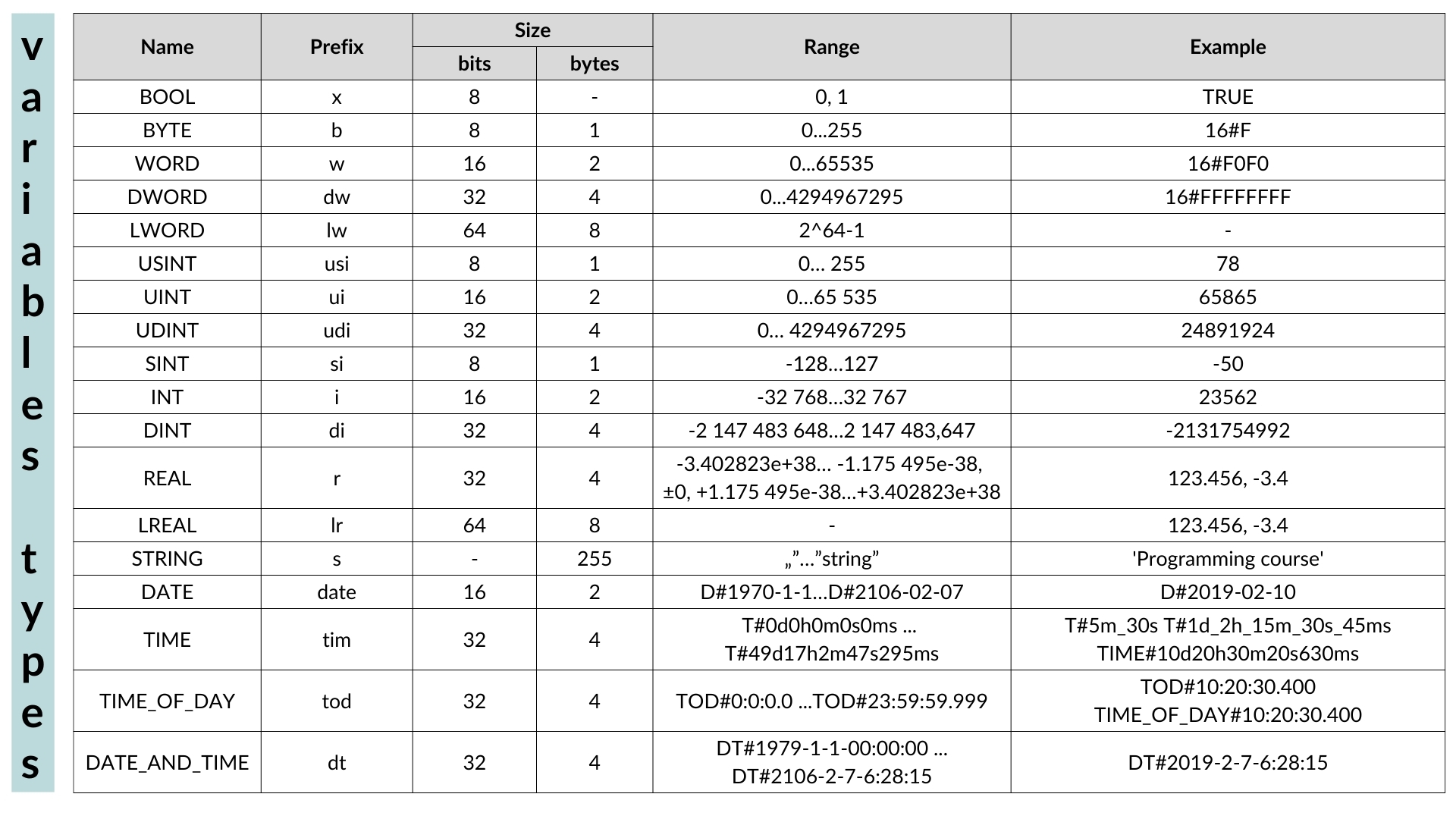
Memory Allocation and Limits
Each integer data type has a different memory size and range. For example:
- Signed Integer (INT): Uses 16 bits and ranges from -32,768 to 32,767.
- Unsigned Integer (UINT): Uses 16 bits but only allows values from 0 to 65,535.
- Signed Byte (SINT): Uses 8 bits and has a range of -128 to 127.
- Unsigned Byte (USINT): Uses 8 bits with a range of 0 to 255.
This means that choosing the appropriate integer type is critical to avoiding data overflow and optimizing memory usage in your PLC programs.
Declaring Integer Variables in CODESYS
Step 1: Opening a Project
- Open CODESYS and create a new project.
- Navigate to PLC_PRG.
Step 2: Declaring Integer Variables
To declare an integer variable, follow these steps:
- Find the prefix for integer variables in the reference table.
- Use the Hungarian notation to name the variable, for example: iVar := 5000;
- i represents an integer variable.
- Var is the chosen name.
- Use := to assign a value, followed by a semicolon (;).
- Press Enter to open the Auto Declare window.
Step 3: Setting the Data Type
- In the Auto Declare window, set the data type to INT.
- The variable’s memory location and scope (local/global) will be determined automatically.
- Click OK to confirm the declaration.
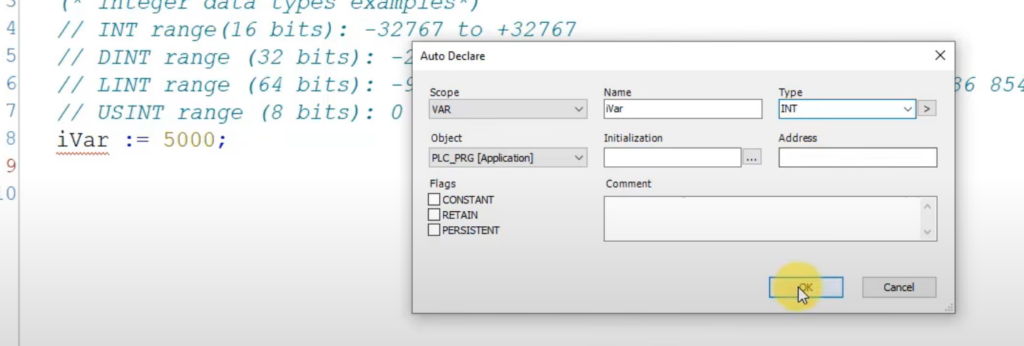
xVar := TRUE; // writing True value to boolean variable
(* Integer data types examples*)
// INT range(16 bits): -32767 to +32767
// DINT range (32 bits): -2 147 483_648 to +2 147 483 647
// LINT range (64 bits): -9 223 372 036 854 775 808 to +9 223 372 036 854 775_807
// USINT range (8 bits): 0 to 255
iVar := 5000;
Handling Integer Overflow
What happens if you exceed the maximum value for an integer type? Let’s test it:
- Declare an integer variable with a value exceeding 32,767: iVar := 40000;
- Click Compile. You will receive an error indicating that the value exceeds the integer range.
- To resolve this, change the data type to DINT (Double Integer): diVar := 40000;
- Also, update the prefix from i to di.
By using DINT, the program will now support a larger value range without errors.
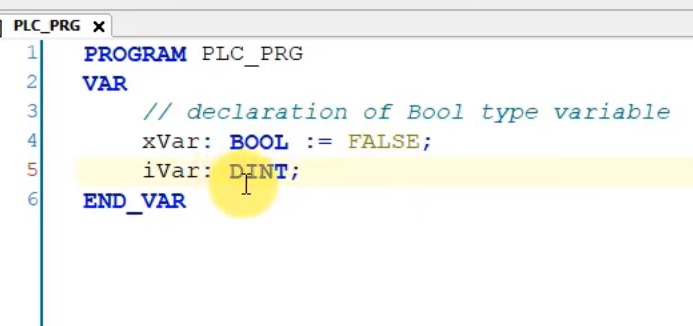
Declaring Unsigned Integer Variables
Unsigned integer variables do not allow negative values. Let’s declare an Unsigned Short Integer (USINT):
- Open the Declaration Window and enter: usiVar : USINT := 200;
- This variable can store values between 0 and 255.
- If you attempt to assign -10, a warning will appear in the compiler: usiVar := -10;
- The compiler will notify you of an implicit conversion issue, as negative values are not allowed.
- Running the simulation will show that the stored value is interpreted differently due to the unsigned limitation.
Optimizing Memory Usage
Why not always use large integer types? The reason is memory efficiency. For example:
- SINT (8-bit) uses less memory than INT (16-bit).
- UINT (16-bit) is more efficient than DINT (32-bit) for storing small positive numbers.
- If memory is well-optimized, PLC programs execute faster, which is critical in automation.
Using integer types wisely ensures efficient memory management and enhances the performance of industrial applications.
Summary
Efficient memory and variable management in CODESYS is crucial for optimizing PLC resources and ensuring proper program execution. This article covers fundamental memory units, variable types, and declaration methods, highlighting the importance of selecting appropriate data types for system performance and stability.
Free PLC course outline
- Lesson #1: What Is It and Why Should Every PLC Programmer Know It? Part #1
- Lesson #2: How to install Codesys? Part #2
- Lesson #3: Write your first program in Codesys: Structured Text – Part #3
- Lesson #4: How to Create a Codesys Visualization in an Application? – Part #4
- Lesson #5: Introduction to Variables in CODESYS – Part #5
- Lesson #6: Data Structures in CODESYS: Practical Use of Arrays and Structures – Part #6